在stm32中,使用HAL_UARTEx_RxEventCallback接受不定长字符串,当串口接受到“blink”时执行闪烁灯,接收到“flow”时执行流水灯,给出代码示例
时间: 2024-10-13 22:09:43 浏览: 64
在STM32中,使用HAL库的UART接收事件回调函数`HAL_UARTEx_RxEventCallback`来监听特定字符串并相应地控制灯的操作,首先需要安装HAL库并理解基本的串口通信流程。以下是一个简单的示例代码,展示了如何实现这个需求:
```c
#include "stm32f1xx_hal.h"
#include "string.h"
typedef enum {
ACTION_BLINK,
ACTION_FLOW,
} Action;
static Action action = ACTION_BLINK;
static uint8_t string_buffer[100];
static volatile bool string_received = false;
// UART HAL Callback function
void HAL_UART_RxCpltCallback(UART_HandleTypeDef *huart) {
if (string_received) {
HAL_UART_Receive_IT(huart, string_buffer, sizeof(string_buffer));
}
}
// Custom Rx Event Callback
void HAL_UARTEx_RxEventCallback(UART_HandleTypeDef *huart, UART_HandleTypeDef__ReceiverInitTypeDef* recv_init_type) {
if (!string_received && strcmp((char*)recv_init_type->pData, "blink")) {
return;
}
string_received = true;
if (strcmp((char*)recv_init_type->pData, "blink")) {
action = ACTION_BLINK;
} else if (strcmp((char*)recv_init_type->pData, "flow")) {
action = ACTION_FLOW;
} else {
// Reset the buffer and received flag when we find an unknown command
memset(string_buffer, 0, sizeof(string_buffer));
string_received = false;
return;
}
// Call your blinking or flow control function here based on the 'action'
handle_light_action(action);
}
// Function to handle light actions based on the received string
void handle_light_action(Action action) {
switch (action) {
case ACTION_BLINK:
// Implement code for blinking light here
break;
case ACTION_FLOW:
// Implement code for flowing light here
break;
default:
break;
}
}
int main(void) {
// Initialize UART and set callbacks
// ...
// Enable RX interrupt and start listening
HAL_UART_Receive_IT(&huart1, string_buffer, sizeof(string_buffer));
while (1) {
// Your main loop can continue here without blocking on UART reception
}
}
阅读全文
相关推荐
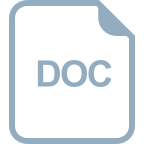
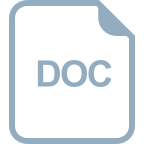
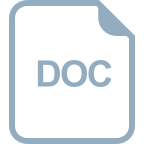




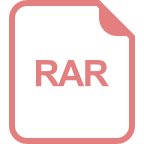
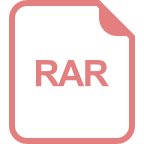
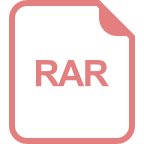
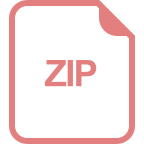
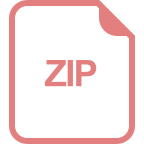
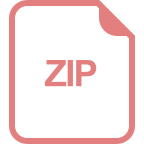