springboor前后端分片上传代码实现
时间: 2024-03-12 10:47:31 浏览: 26
Spring Boot提供了一种方便的方式来实现前后端分片上传,可以通过以下步骤进行操作:
1. 前端代码实现
在前端代码中,需要使用HTML5的File API来实现文件的切片和上传,然后将切片数据发送到后端进行处理。
以下是一个示例代码:
```javascript
// 文件切片大小
const chunkSize = 1024 * 1024;
// 文件切片数量
let chunkCount = 0;
// 当前上传的切片编号
let currentChunk = 0;
// 上传的文件对象
let file = null;
// 分片上传
function upload() {
// 读取文件
const reader = new FileReader();
reader.onload = function(event) {
// 上传切片数据
const chunkData = event.target.result;
const formData = new FormData();
formData.append('file', file.name);
formData.append('chunk', currentChunk);
formData.append('data', chunkData);
axios.post('/api/upload', formData)
.then(response => {
// 上传成功,继续上传下一个切片
currentChunk++;
if (currentChunk < chunkCount) {
upload();
} else {
// 所有切片上传完成
console.log('Upload success');
}
})
.catch(error => {
console.error('Upload failed', error);
});
};
// 读取切片数据
const start = currentChunk * chunkSize;
const end = Math.min((currentChunk + 1) * chunkSize, file.size);
reader.readAsArrayBuffer(file.slice(start, end));
}
// 文件选择
function selectFile(event) {
file = event.target.files[0];
chunkCount = Math.ceil(file.size / chunkSize);
currentChunk = 0;
}
```
2. 后端代码实现
在后端代码中,需要实现上传接口,根据前端上传的切片数据,将数据合并为完整的文件。
以下是一个示例代码:
```java
@RestController
@RequestMapping("/api")
public class UploadController {
// 上传切片
@PostMapping("/upload")
public void upload(MultipartFile file, Integer chunk, String data) throws IOException {
// 切片文件存储路径
Path chunkPath = Paths.get("upload", file.getOriginalFilename(), chunk.toString());
// 写入切片文件
Files.write(chunkPath, Base64.getDecoder().decode(data));
}
// 合并文件
@PostMapping("/merge")
public void merge(String filename) throws IOException {
// 完整文件存储路径
Path filePath = Paths.get("upload", filename);
// 获取所有切片文件
List<Path> chunkPaths = Files.list(Paths.get("upload", filename))
.filter(path -> !Files.isDirectory(path))
.sorted()
.collect(Collectors.toList());
// 合并切片文件
try (OutputStream outputStream = Files.newOutputStream(filePath)) {
for (Path chunkPath : chunkPaths) {
Files.copy(chunkPath, outputStream);
Files.delete(chunkPath);
}
}
}
}
```
在上传完成后,可以通过调用`/api/merge`接口将所有切片合并为完整的文件。
同时,还需要在应用启动时创建存储切片和文件的目录:
```java
@SpringBootApplication
public class Application implements CommandLineRunner {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Override
public void run(String... args) throws Exception {
Files.createDirectories(Paths.get("upload"));
}
}
```
这样,就完成了前后端分片上传的代码实现。
相关推荐
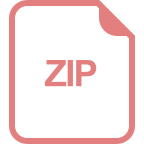
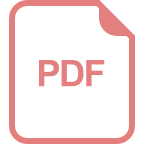
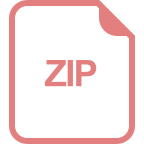
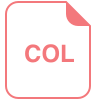
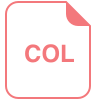
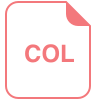
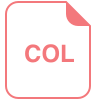
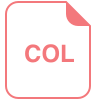









