pythonrsa加解密txt文件并实现图形化ui界面
时间: 2023-08-05 21:18:30 浏览: 60
要实现PythonRSA加解密txt文件并实现图形化UI界面,可以按照以下步骤进行:
1. 安装PythonRSA库
可以使用pip命令安装PythonRSA库,命令如下:
```python
pip install python-rsa
```
2. 编写加密解密脚本
可以使用PythonRSA库提供的RSA算法进行加密解密。以下是一个简单的加解密脚本示例:
```python
import rsa
# 生成RSA密钥对
(pubkey, privkey) = rsa.newkeys(512)
# 加密
message = 'Hello, world!'
crypto = rsa.encrypt(message.encode(), pubkey)
# 解密
message = rsa.decrypt(crypto, privkey).decode()
print(message)
```
3. 编写图形化UI界面
可以使用Python的GUI库Tkinter来实现图形化界面。以下是一个简单的界面示例:
```python
import tkinter as tk
import rsa
# 生成RSA密钥对
(pubkey, privkey) = rsa.newkeys(512)
def encrypt():
message = plaintext.get()
crypto = rsa.encrypt(message.encode(), pubkey)
ciphertext.delete(0, tk.END)
ciphertext.insert(0, crypto.hex())
def decrypt():
crypto = bytes.fromhex(ciphertext.get())
message = rsa.decrypt(crypto, privkey).decode()
plaintext.delete(0, tk.END)
plaintext.insert(0, message)
root = tk.Tk()
root.title('PythonRSA加解密')
# 明文输入框
tk.Label(root, text='明文:').grid(row=0, column=0)
plaintext = tk.Entry(root)
plaintext.grid(row=0, column=1)
# 密文输入框
tk.Label(root, text='密文:').grid(row=1, column=0)
ciphertext = tk.Entry(root)
ciphertext.grid(row=1, column=1)
# 加密按钮
encrypt_button = tk.Button(root, text='加密', command=encrypt)
encrypt_button.grid(row=2, column=0)
# 解密按钮
decrypt_button = tk.Button(root, text='解密', command=decrypt)
decrypt_button.grid(row=2, column=1)
root.mainloop()
```
这个界面中,有一个明文输入框和一个密文输入框,分别用于输入明文和密文;有一个加密按钮和一个解密按钮,分别用于进行加密和解密操作。加解密操作通过调用前面编写的加解密脚本实现。
相关推荐
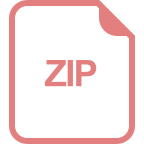
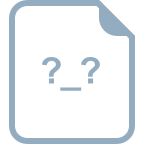














