tensorflow2 CNN模型验证码识别
时间: 2023-09-03 22:15:33 浏览: 57
验证码识别是一个常见的图像识别问题,卷积神经网络(CNN)是其中一种常用的解决方案。下面是一个使用 TensorFlow 2 实现的简单 CNN 模型来识别验证码的例子:
首先,导入必要的库:
```python
import os
import numpy as np
import tensorflow as tf
from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
```
接着,定义一些超参数:
```python
IMG_WIDTH = 60
IMG_HEIGHT = 20
BATCH_SIZE = 32
EPOCHS = 10
NUM_CLASSES = 4
```
其中,`IMG_WIDTH` 和 `IMG_HEIGHT` 分别代表输入图像的宽度和高度,`BATCH_SIZE` 是每次训练时使用的样本数量,`EPOCHS` 是训练迭代次数,`NUM_CLASSES` 是验证码字符集的大小。
然后,准备数据集。假设我们有一个包含 1000 张验证码图片的数据集,每张图片都是 60x20 的灰度图像,保存在 `data` 文件夹下,文件名为 `captcha_{i}.png`(`i` 从 1 到 1000)。我们需要将数据集分成训练集和测试集,并使用 `ImageDataGenerator` 类来对图像进行预处理:
```python
train_datagen = ImageDataGenerator(
rescale=1./255,
shear_range=0.2,
zoom_range=0.2,
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
validation_split=0.2)
train_generator = train_datagen.flow_from_directory(
'data',
target_size=(IMG_HEIGHT, IMG_WIDTH),
batch_size=BATCH_SIZE,
color_mode='grayscale',
class_mode='categorical',
subset='training')
test_generator = train_datagen.flow_from_directory(
'data',
target_size=(IMG_HEIGHT, IMG_WIDTH),
batch_size=BATCH_SIZE,
color_mode='grayscale',
class_mode='categorical',
subset='validation')
```
其中,`train_datagen` 定义了一系列图像增强操作,包括缩放、剪切、旋转、平移等。`train_generator` 和 `test_generator` 分别是训练集和测试集的生成器。
接下来,构建 CNN 模型:
```python
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(IMG_HEIGHT, IMG_WIDTH, 1)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Conv2D(128, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dropout(0.5),
Dense(512, activation='relu'),
Dropout(0.5),
Dense(NUM_CLASSES, activation='softmax')
])
```
该模型包含 3 个卷积层、3 个池化层和 2 个全连接层,其中每个卷积层后面都跟着一个最大池化层。最后一层是一个大小为 `NUM_CLASSES` 的 softmax 层,用于分类。
最后,编译模型并开始训练:
```python
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(train_generator, epochs=EPOCHS, validation_data=test_generator)
```
训练完成后,我们可以使用模型来预测新的验证码图片:
```python
def predict_captcha(filename):
img = tf.keras.preprocessing.image.load_img(filename, color_mode='grayscale', target_size=(IMG_HEIGHT, IMG_WIDTH))
img = tf.keras.preprocessing.image.img_to_array(img)
img = np.expand_dims(img, axis=0)
img /= 255.
prediction = model.predict(img)
prediction = np.argmax(prediction, axis=1)
return prediction[0]
```
该函数接受一个验证码图片的文件名,返回模型预测出的验证码字符的标签。
以上就是使用 TensorFlow 2 实现验证码识别的一个简单例子。
相关推荐
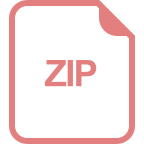
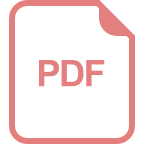














