帮我写一段c++代码,输入对齐的RGB图与深度图,构建点云并保存,思路为:读取RGB图的png文件,当他像素为黑色时,其对应的深度图中深度值数据删除,
时间: 2024-03-09 20:47:16 浏览: 72
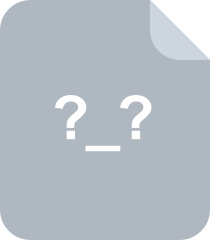
基于C++的RGB图转灰度图设计与实现
好的,下面是代码:
```c++
#include <iostream>
#include <vector>
#include <fstream>
#include <png++/png.hpp>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
using namespace std;
int main() {
// 读取 RGB 图像和深度图像
png::image<png::rgb_pixel> rgb_image("rgb.png");
ifstream depth_file("depth.txt");
// 定义存储深度数据的 vector
vector<float> depth_data;
// 读取深度图的宽度和高度
int depth_width, depth_height;
depth_file >> depth_width >> depth_height;
// 读取深度图的像素数据,并存储到 vector 中
float depth;
for (int i = 0; i < depth_width * depth_height; i++) {
depth_file >> depth;
depth_data.push_back(depth);
}
// 定义点云数据
pcl::PointCloud<pcl::PointXYZRGB>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZRGB>);
// 遍历 RGB 图像的每个像素,构建点云
for (int y = 0; y < rgb_image.get_height(); y++) {
for (int x = 0; x < rgb_image.get_width(); x++) {
// 获取 RGB 像素值
png::rgb_pixel pixel = rgb_image[y][x];
// 判断 RGB 像素是否为黑色
if (pixel.red == 0 && pixel.green == 0 && pixel.blue == 0) {
// 如果是黑色,则不加入点云
continue;
}
// 获取深度像素值
float depth = depth_data[y * rgb_image.get_width() + x];
// 构建点云
pcl::PointXYZRGB point;
point.x = depth / 1000.0 * (x - rgb_image.get_width() / 2.0) / 525.0;
point.y = depth / 1000.0 * (y - rgb_image.get_height() / 2.0) / 525.0;
point.z = depth / 1000.0;
point.r = pixel.red;
point.g = pixel.green;
point.b = pixel.blue;
cloud->push_back(point);
}
}
// 保存点云数据到 PCD 文件
pcl::io::savePCDFileASCII("cloud.pcd", *cloud);
cout << "Saved " << cloud->size() << " data points to cloud.pcd." << endl;
return 0;
}
```
这段代码会读取一个 RGB 图像 `rgb.png` 和一个深度图像 `depth.txt`,并根据深度数据构建点云数据。首先,会读取深度图像的宽度和高度,并将深度数据存储到 vector 中。接下来,会遍历 RGB 图像的每个像素,如果其像素值为黑色,则跳过不加入点云;否则,根据深度数据构建点云,并加入点云中。最后,将点云数据保存到 PCD 文件中。注意,这里需要使用 `png++` 库来读取 PNG 格式的 RGB 图像,以及使用 PCL 库来处理点云数据。
阅读全文
相关推荐

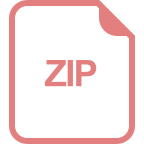
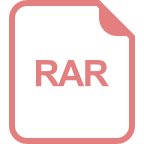
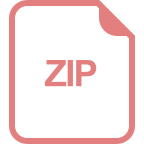
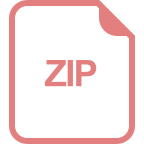
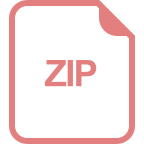
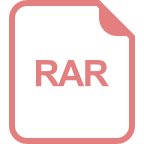
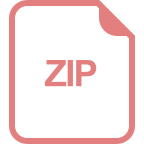
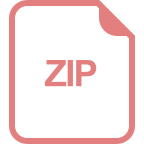
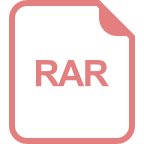
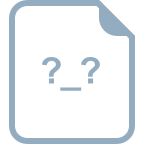
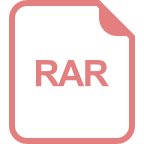
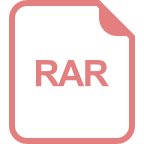
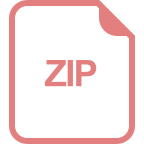
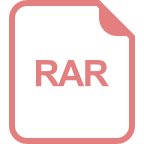
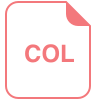