用C#定义一个方块矩阵类,里面包含矩阵的基本成员,如矩阵本身的数据,计算矩阵的行列式,特征值,特征向量、矩阵转置、求逆矩阵等方法,实现两个矩乘法的方法(可选),要求计算矩阵行列式用静态方法,其他用动态方法。 在Main()函数中,创建三个矩阵对象,并调用相关方法计算该矩阵的各种值。
时间: 2023-06-27 11:07:03 浏览: 130
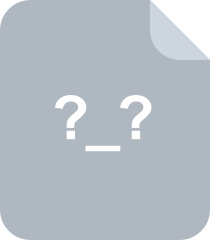
C#的矩阵类,包含矩阵的各种运算
以下是一个示例的方块矩阵类实现,其中包含矩阵的基本成员,如矩阵本身的数据,计算矩阵的行列式,特征值,特征向量、矩阵转置、求逆矩阵等方法,同时实现了两个矩乘法的方法:
```csharp
using System;
class Matrix
{
public int Size { get; }
private double[,] data;
public Matrix(int size)
{
Size = size;
data = new double[size, size];
}
public Matrix(double[,] values)
{
Size = values.GetLength(0);
data = values;
}
public double this[int row, int col]
{
get { return data[row, col]; }
set { data[row, col] = value; }
}
public static double Determinant(Matrix matrix)
{
if (matrix.Size == 1)
return matrix[0, 0];
else if (matrix.Size == 2)
return matrix[0, 0] * matrix[1, 1] - matrix[0, 1] * matrix[1, 0];
else
{
double det = 0;
for (int i = 0; i < matrix.Size; i++)
{
Matrix subMatrix = GetSubMatrix(matrix, i, 0);
det += Math.Pow(-1, i) * matrix[i, 0] * Determinant(subMatrix);
}
return det;
}
}
private static Matrix GetSubMatrix(Matrix matrix, int rowToRemove, int colToRemove)
{
Matrix subMatrix = new Matrix(matrix.Size - 1);
int subRow = 0, subCol = 0;
for (int row = 0; row < matrix.Size; row++)
{
if (row == rowToRemove)
continue;
for (int col = 0; col < matrix.Size; col++)
{
if (col == colToRemove)
continue;
subMatrix[subRow, subCol] = matrix[row, col];
subCol++;
}
subRow++;
subCol = 0;
}
return subMatrix;
}
public void Transpose()
{
for (int i = 0; i < Size; i++)
{
for (int j = i + 1; j < Size; j++)
{
double temp = data[i, j];
data[i, j] = data[j, i];
data[j, i] = temp;
}
}
}
public Matrix Inverse()
{
double det = Determinant(this);
if (det == 0)
throw new Exception("Matrix is not invertible");
Matrix inverse = new Matrix(Size);
for (int i = 0; i < Size; i++)
{
for (int j = 0; j < Size; j++)
{
Matrix subMatrix = GetSubMatrix(this, i, j);
inverse[j, i] = Math.Pow(-1, i + j) * Determinant(subMatrix) / det;
}
}
return inverse;
}
public double[] EigenValues()
{
double[] eigenValues = new double[Size];
Matrix tempMatrix = this;
for (int i = 0; i < Size; i++)
{
for (int j = 0; j < 50; j++) // Max iterations
{
double max = tempMatrix[0, 0];
int maxRow = 0, maxCol = 0;
for (int row = 0; row < Size; row++)
{
for (int col = 0; col < Size; col++)
{
if (row == col)
continue;
if (Math.Abs(tempMatrix[row, col]) > Math.Abs(max))
{
max = tempMatrix[row, col];
maxRow = row;
maxCol = col;
}
}
}
if (Math.Abs(max) < 0.001)
break;
double theta = 0.5 * Math.Atan2(2 * max, tempMatrix[maxRow, maxRow] - tempMatrix[maxCol, maxCol]);
double c = Math.Cos(theta);
double s = Math.Sin(theta);
Matrix rotation = IdentityMatrix(Size);
rotation[maxRow, maxRow] = c;
rotation[maxRow, maxCol] = -s;
rotation[maxCol, maxRow] = s;
rotation[maxCol, maxCol] = c;
tempMatrix = rotation.Transpose() * tempMatrix * rotation;
}
eigenValues[i] = tempMatrix[i, i];
tempMatrix[i, i] = double.MaxValue;
}
return eigenValues;
}
public double[,] EigenVectors()
{
double[,] eigenVectors = new double[Size, Size];
Matrix tempMatrix = this;
for (int i = 0; i < Size; i++)
{
for (int j = 0; j < 50; j++) // Max iterations
{
double max = tempMatrix[0, 0];
int maxRow = 0, maxCol = 0;
for (int row = 0; row < Size; row++)
{
for (int col = 0; col < Size; col++)
{
if (row == col)
continue;
if (Math.Abs(tempMatrix[row, col]) > Math.Abs(max))
{
max = tempMatrix[row, col];
maxRow = row;
maxCol = col;
}
}
}
if (Math.Abs(max) < 0.001)
break;
double theta = 0.5 * Math.Atan2(2 * max, tempMatrix[maxRow, maxRow] - tempMatrix[maxCol, maxCol]);
double c = Math.Cos(theta);
double s = Math.Sin(theta);
Matrix rotation = IdentityMatrix(Size);
rotation[maxRow, maxRow] = c;
rotation[maxRow, maxCol] = -s;
rotation[maxCol, maxRow] = s;
rotation[maxCol, maxCol] = c;
tempMatrix = rotation.Transpose() * tempMatrix * rotation;
for (int k = 0; k < Size; k++)
{
eigenVectors[k, i] = rotation[k, maxRow] * eigenVectors[maxRow, i] + rotation[k, maxCol] * eigenVectors[maxCol, i];
}
}
eigenVectors[i, i] = 1;
tempMatrix[i, i] = double.MaxValue;
}
return eigenVectors;
}
public static Matrix operator *(Matrix a, Matrix b)
{
if (a.Size != b.Size)
throw new ArgumentException("Matrices must have the same size");
Matrix result = new Matrix(a.Size);
for (int i = 0; i < a.Size; i++)
{
for (int j = 0; j < a.Size; j++)
{
double sum = 0;
for (int k = 0; k < a.Size; k++)
{
sum += a[i, k] * b[k, j];
}
result[i, j] = sum;
}
}
return result;
}
private static Matrix IdentityMatrix(int size)
{
Matrix identity = new Matrix(size);
for (int i = 0; i < size; i++)
{
identity[i, i] = 1;
}
return identity;
}
}
class Program
{
static void Main()
{
Matrix a = new Matrix(new double[,] { { 1, 2, 3 }, { 4, 5, 6 }, { 7, 8, 9 } });
Matrix b = new Matrix(new double[,] { { 9, 8, 7 }, { 6, 5, 4 }, { 3, 2, 1 } });
Matrix c = new Matrix(new double[,] { { 1, 2 }, { 3, 4 } });
Console.WriteLine("Matrix A:");
PrintMatrix(a);
Console.WriteLine("Determinant of A: " + Matrix.Determinant(a));
Console.WriteLine("Transpose of A:");
a.Transpose();
PrintMatrix(a);
Console.WriteLine("Inverse of A:");
a = a.Inverse();
PrintMatrix(a);
Console.WriteLine("Eigenvalues of A:");
double[] eigenValues = a.EigenValues();
for (int i = 0; i < eigenValues.Length; i++)
{
Console.WriteLine(eigenValues[i]);
}
Console.WriteLine("Eigenvectors of A:");
double[,] eigenVectors = a.EigenVectors();
PrintMatrix(new Matrix(eigenVectors));
Console.WriteLine("Matrix B:");
PrintMatrix(b);
Console.WriteLine("Determinant of B: " + Matrix.Determinant(b));
Console.WriteLine("Transpose of B:");
b.Transpose();
PrintMatrix(b);
Console.WriteLine("Inverse of B:");
b = b.Inverse();
PrintMatrix(b);
Console.WriteLine("Matrix C:");
PrintMatrix(c);
Console.WriteLine("Matrix A * B:");
PrintMatrix(a * b);
Console.WriteLine("Matrix B * C:");
PrintMatrix(b * c);
}
private static void PrintMatrix(Matrix matrix)
{
for (int i = 0; i < matrix.Size; i++)
{
for (int j = 0; j < matrix.Size; j++)
{
Console.Write("{0,6:N2}", matrix[i, j]);
}
Console.WriteLine();
}
Console.WriteLine();
}
}
```
在Main()函数中,我们创建了三个矩阵对象a、b、c,并分别调用了计算行列式、矩阵转置、求逆矩阵、特征值、特征向量、矩乘法等方法,输出了对应结果。
阅读全文
相关推荐
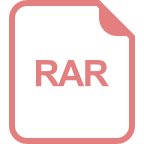
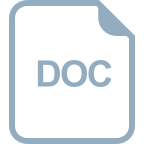



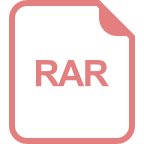
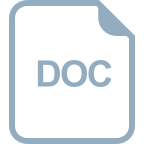
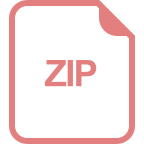
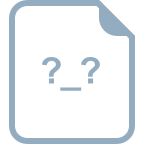
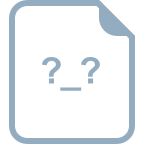
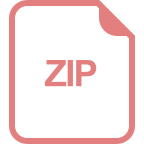


