python生日快乐粒子代码
时间: 2024-12-18 20:11:20 浏览: 6
Python中创建一个生日快乐的粒子动画效果,可以使用pygame库结合循环和图像处理来实现。这是一个简单的例子:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置窗口大小
screen_width = 800
screen_height = 600
win = pygame.display.set_mode((screen_width, screen_height))
# 生日快乐文字
happy_birthday_surface = pygame.font.SysFont("arial", 70).render("生日快乐!", True, (255, 255, 255))
# 颜色列表
colors = [(random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)) for _ in range(100)]
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
self.speed = [random.uniform(-3, 3) for _ in range(2)]
self.color = colors.pop()
def update(self):
self.x += self.speed[0]
self.y += self.speed[1]
if self.x < 0 or self.x > screen_width or self.y < 0 or self.y > screen_height:
self.speed = [-self.speed[0], -self.speed[1]]
def draw(self):
pygame.draw.circle(win, self.color, (int(self.x), int(self.y)), 5)
# 创建粒子集合
particles = [Particle(screen_width // 2, screen_height // 2) for _ in range(100)]
# 游戏主循环
running = True
clock = pygame.time.Clock()
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
win.fill((0, 0, 0))
# 更新并绘制粒子
for particle in particles:
particle.update()
particle.draw()
# 绘制"生日快乐"
win.blit(happy_birthday_surface, (0, 0))
# 更新屏幕
pygame.display.flip()
clock.tick(30) # 控制帧率
# 结束游戏
pygame.quit()
```
在这个代码中,我们首先导入了必要的库,然后定义了一个粒子类(Particle),包含了位置、速度和颜色属性,并实现了更新和绘制方法。接着创建了一组随机位置的粒子,并在一个无限循环中不断更新它们的位置和绘制到屏幕上。同时,还有一个"生日快乐"的文字显示在窗口顶部。
阅读全文
相关推荐
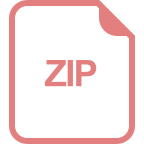
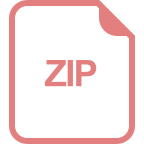
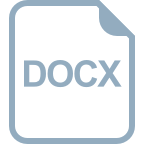
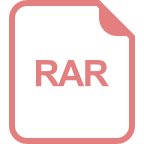
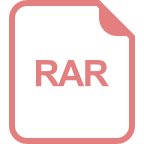
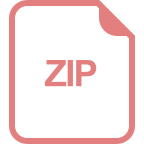
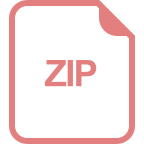
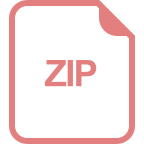
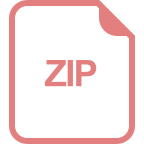
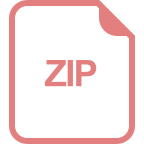
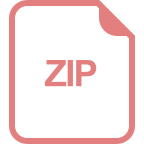
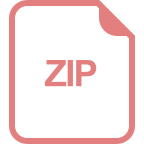