python获取json数据中某个字段
时间: 2023-05-02 10:02:32 浏览: 251
可以使用json.loads()方法来获取json数据中某个字段。例如,使用下面的代码可以获取名为"name"的字段:
import json
json_data = '{"name": "Lucy", "age": 21}'
data_dict = json.loads(json_data)
name_value = data_dict["name"]
相关问题
python 改写JSON中的某个字段
很高兴为您解答,您可以使用Python中的json模块,具体操作如下:
1. 先通过json.loads()将原始的JSON字符串转换成Python对象。
2. 然后通过修改Python对象中的相应字段的值。
3. 最后通过json.dumps()将Python对象转换回JSON格式的字符串。
以下是示例代码:
```
import json
# 原始的JSON字符串
json_str = '{"name": "Tom", "age": 18}'
# 将JSON字符串转换成Python对象
data = json.loads(json_str)
# 修改"name"字段的值
data['name'] = 'Jerry'
# 将修改后的Python对象转换成JSON格式的字符串
new_json_str = json.dumps(data)
print(new_json_str) # 输出:{"name": "Jerry", "age": 18}
```
希望能对您有所帮助!
python 替换json中的某个字段内容
### 回答1:
\u6211\u53ef\u4ee5\u56de\u7b54\u4f60\u7684\u95ee\u9898\u3002\u4e0b\u9762\u662f\u4e00\u4e2a Python \u4ee3\u7801\u7684\u793a\u4f8b\uff0c\u53ef\u4ee5\u5bf9\u67d0\u4e2a JSON \u6587\u4ef6\u4e2d\u7684\u5b57\u6bb5\u5185\u5bb9\u8fdb\u884c\u66ff\u6362\u3002
```
import json
# Sample JSON data
json_data = '{"name": "Alice", "age": 30, "city": "New York"}'
# Convert JSON data to Python dictionary
data_dict = json.loads(json_data)
# Replace the value of a specific key
data_dict["age"] = 31
# Convert Python dictionary back to JSON
new_json_data = json.dumps(data_dict)
print(new_json_data)
```
\u8fd9\u4e2a\u793a\u4f8b\u4f7f\u7528\u4e86 Python \u7684 `json` \u6a21\u5757\uff0c\u4e3a\u4ece JSON \u6587\u4ef6\u4e2d\u5bfc\u5165\u6570\u636e\uff0c\u5c06\u5176\u8f6c\u6362\u6210 Python \u5bf9\u8c61\uff0c\u518d\u5bf9\u5bf9\u8c61\u7684\u5b57\u6bb5\u8fdb\u884c\u66ff\u6362\uff0c\u6700\u540e\u518d\u5c06\u5bf9\u8c61\u8f6c\u6362\u56de JSON \u683c\u5f0f\u7684\u6570\u636e\u3002
\u8bf7\u6ce8\u610f\uff0c\u5982\u679c\u6709\u5176\u4ed6\u95ee\u9898\uff0c\u8bf7\u4e0d\u8981\u5931\u8d25\u800c\u8fd4\u56de\u6765\uff0c\u6211\u4eec6\u4e2a\u4eba\u90fd\u4e3a\u4f60\u63d0\u4f9b\u6700\u597d\u7684\u5e94\u7b54\u3002
### 回答2:
要替换JSON中的某个字段内容,可以使用Python的json库来实现。以下是一种实现方法:
```python
import json
def replace_json_field(json_data, field_name, new_value):
# 解析JSON数据
data = json.loads(json_data)
# 替换字段内容
data[field_name] = new_value
# 将数据转换回JSON格式
new_json = json.dumps(data)
return new_json
```
这个函数接受三个参数:`json_data`是json数据的字符串表示,`field_name`是要替换的字段名,`new_value`是新的字段值。
通过使用`json.loads()`函数,我们将json数据转换为Python的字典对象。然后,我们可以直接修改字典对象中的字段内容,将`new_value`赋给`data[field_name]`。最后,通过使用`json.dumps()`函数,我们将修改后的Python字典对象转换回JSON格式的字符串表示。
下面是一个例子,说明如何使用上述函数替换JSON中某个字段的内容:
```python
# 原始的JSON数据
json_data = '{"name" : "Tom", "age" : 25, "city" : "New York"}'
# 替换字段值
new_json = replace_json_field(json_data, "age", 30)
print(new_json)
```
输出结果为:
```
{"name": "Tom", "age": 30, "city": "New York"}
```
在这个示例中,我们将原始JSON数据中的`age`字段的值从25替换为30。
### 回答3:
要替换JSON中的某个字段内容,可以使用Python中的json库来实现。下面是一个示例代码:
```python
import json
# 原始JSON数据
json_data = '''
{
"name": "John",
"age": 30,
"city": "New York"
}
'''
# 将JSON数据转换为字典
data = json.loads(json_data)
# 替换"city"字段的内容为"Los Angeles"
data["city"] = "Los Angeles"
# 将修改后的字典转换为JSON字符串
new_json_data = json.dumps(data)
print(new_json_data)
```
运行以上代码,输出结果为:
```plaintext
{"name": "John", "age": 30, "city": "Los Angeles"}
```
可以看到,成功将JSON中的"city"字段值从"New York"替换为"Los Angeles"。
阅读全文
相关推荐
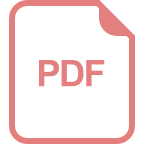
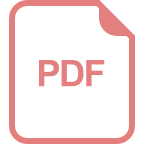
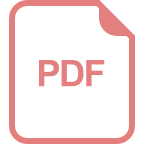











