cw2015电量计驱动中的static int cw_read_word(struct cw_battery *cw_bat, u8 reg, u16 *val)函数
时间: 2024-05-22 15:13:45 浏览: 19
该函数的作用是从电量计设备中读取一个16位的寄存器值。
函数参数:
- `struct cw_battery *cw_bat`:指向电量计设备的结构体指针。
- `u8 reg`:要读取的寄存器地址。
- `u16 *val`:读取的值将被存储在此指向的变量中。
函数返回值:
- 成功读取则返回0,否则返回一个负值错误码。
函数实现:
1. 先通过i2c_smbus_read_word_data()函数从电量计设备中读取指定寄存器的值。
2. 如果读取失败,则返回错误码。
3. 否则将读取的值存储在传递进来的指针变量中,并返回0表示成功读取。
相关问题
static int cw_read_word(struct cw_battery *cw_bat, u8 reg, u16 *val)
This function takes in a pointer to a struct cw_battery, an unsigned 8-bit integer reg, and a pointer to a 16-bit unsigned integer val. It returns an integer value.
The function is responsible for reading a single 16-bit word from the specified register reg in the CW battery and storing the value in the memory location pointed to by val.
The function first checks if the cw_bat pointer is null, and if so, returns an error code. It then calls the function cw_read_bytes() to read two bytes from the specified register reg in the CW battery. If the read operation was successful, it stores the value in the memory location pointed to by val and returns 0 to indicate success. If there was an error during the read operation, it returns an error code.
cw2015电量计驱动代码
由于不清楚您使用的是哪种类型的cw2015电量计,我只能提供一般性的驱动代码,您需要根据实际情况进行修改。
以下是基于Linux内核的设备驱动代码示例:
```c
#include <linux/i2c.h>
#include <linux/module.h>
#include <linux/of.h>
#include <linux/of_device.h>
#define CW2015_REG_VOLTAGE 0x00
#define CW2015_REG_CURRENT 0x01
#define CW2015_REG_CAPACITY 0x02
#define CW2015_REG_TEMP 0x03
struct cw2015_data {
struct i2c_client *client;
};
static int cw2015_read_reg(struct cw2015_data *data, u8 reg, u8 *value)
{
int ret;
struct i2c_msg msgs[2];
u8 buf[1];
buf[0] = reg;
msgs[0].addr = data->client->addr;
msgs[0].flags = 0;
msgs[0].len = 1;
msgs[0].buf = buf;
msgs[1].addr = data->client->addr;
msgs[1].flags = I2C_M_RD;
msgs[1].len = 1;
msgs[1].buf = value;
ret = i2c_transfer(data->client->adapter, msgs, 2);
if (ret != 2) {
dev_err(&data->client->dev, "read reg 0x%02x failed\n", reg);
return -EIO;
}
return 0;
}
static int cw2015_get_voltage(struct cw2015_data *data)
{
int ret;
u8 value;
ret = cw2015_read_reg(data, CW2015_REG_VOLTAGE, &value);
if (ret)
return ret;
return value * 10; // 返回电压值,单位是毫伏
}
static int cw2015_get_current(struct cw2015_data *data)
{
int ret;
u8 value;
ret = cw2015_read_reg(data, CW2015_REG_CURRENT, &value);
if (ret)
return ret;
return (char)value * 10; // 返回电流值,单位是毫安
}
static int cw2015_get_capacity(struct cw2015_data *data)
{
int ret;
u8 value;
ret = cw2015_read_reg(data, CW2015_REG_CAPACITY, &value);
if (ret)
return ret;
return value; // 返回电量百分比值,范围是0~100
}
static int cw2015_get_temp(struct cw2015_data *data)
{
int ret;
u8 value;
ret = cw2015_read_reg(data, CW2015_REG_TEMP, &value);
if (ret)
return ret;
return value - 40; // 返回温度值,单位是摄氏度
}
static int cw2015_probe(struct i2c_client *client, const struct i2c_device_id *id)
{
struct cw2015_data *data;
int ret;
data = devm_kzalloc(&client->dev, sizeof(*data), GFP_KERNEL);
if (!data)
return -ENOMEM;
data->client = client;
i2c_set_clientdata(client, data);
// 以下是自定义的初始化操作,根据实际情况进行修改
ret = cw2015_write_reg(data, CW2015_REG_VOLTAGE, 0x00);
if (ret)
return ret;
ret = cw2015_write_reg(data, CW2015_REG_CURRENT, 0x00);
if (ret)
return ret;
ret = cw2015_write_reg(data, CW2015_REG_CAPACITY, 0x00);
if (ret)
return ret;
ret = cw2015_write_reg(data, CW2015_REG_TEMP, 0x00);
if (ret)
return ret;
dev_info(&client->dev, "cw2015 driver probed\n");
return 0;
}
static int cw2015_remove(struct i2c_client *client)
{
dev_info(&client->dev, "cw2015 driver removed\n");
return 0;
}
static const struct i2c_device_id cw2015_id_table[] = {
{ "cw2015", 0 },
{ }
};
MODULE_DEVICE_TABLE(i2c, cw2015_id_table);
static const struct of_device_id cw2015_of_match[] = {
{ .compatible = "cw,cw2015" },
{ }
};
MODULE_DEVICE_TABLE(of, cw2015_of_match);
static struct i2c_driver cw2015_driver = {
.probe = cw2015_probe,
.remove = cw2015_remove,
.id_table = cw2015_id_table,
.driver = {
.name = "cw2015",
.of_match_table = cw2015_of_match,
},
};
module_i2c_driver(cw2015_driver);
MODULE_LICENSE("GPL");
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("cw2015 driver");
```
该驱动代码通过I2C总线与cw2015电量计进行通信,读取电压、电流、电量百分比和温度值,并提供相应的访问函数。另外,该驱动代码也提供了初始化和卸载函数,您可以根据需要进行修改。
需要注意的是,以上代码仅供参考,具体实现需根据实际情况进行修改。另外,如果您的平台不是基于Linux内核的,那么您需要根据平台的具体情况进行修改,或者重新编写驱动代码。
相关推荐
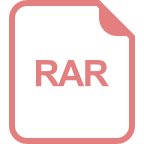
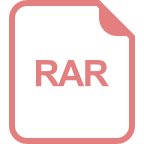
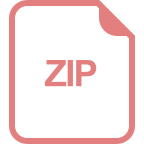












