<?php namespace App\Service\Rule; Interface RuleInterface { function getCartItems(); function setCartItems($cartItems); function collect(); }
时间: 2024-03-29 13:38:26 浏览: 120
这段代码是一个 PHP 命名空间(namespace)为 App\Service\Rule 的代码文件,其中定义了一个名为 RuleInterface 的接口(interface)。这个接口有三个方法:
1. getCartItems():获取购物车中的商品列表。
2. setCartItems($cartItems):设置购物车中的商品列表。
3. collect():计算购物车中商品的总价并返回。
这个接口可能是用于实现购物车价格计算的规则类,其他类可以实现该接口以提供不同的价格计算规则。
相关问题
用java帮我写public class ShoppingCart { private int itemCount; // 购物车中商品数量 private double totalPrice; // 购物车中商品总价钱 private Map<String, Integer> cartItems; // 购物车中商品信息 public ShoppingCart() { itemCount = 0; totalPrice = 0.0; cartItems = new HashMap<String, Integer>(); } // 添加商品到购物车 public void addItem(String productId, int quantity, double price) { if (!cartItems.containsKey(productId)) { cartItems.put(productId, quantity); } else { int qty = cartItems.get(productId); qty += quantity; cartItems.put(productId, qty); } itemCount += quantity; totalPrice += price * quantity; } // 更新购物车中商品数量 public void update(String productId, int quantity, double price) { if (cartItems.containsKey(productId)) { int qty = cartItems.get(productId); itemCount -= qty; totalPrice -= qty * price; cartItems.put(productId, quantity); itemCount += quantity; totalPrice += quantity * price; } } // 删除购物车中的商品 public void removeItem(String productId, double price) { if (cartItems.containsKey(productId)) { int qty = cartItems.get(productId); itemCount -= qty; totalPrice -= qty * price; cartItems.remove(productId); } } // 清空购物车 public void clear() { cartItems.clear(); itemCount = 0; totalPrice = 0.0; } // 获取购物车中商品数量 public int getItemCount() { return itemCount; } // 获取购物车中商品总价钱 public double getTotalPrice() { return totalPrice; } // 获取购物车中的商品信息 public Map<String, Integer> getCartItems() { return cartItems; } }的测试代码
好的,下面是可以用来测试 ShoppingCart 类的 Java 代码:
public class ShoppingCartTest {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
// 添加商品到购物车
cart.addItem("1001", 2, 10.0);
cart.addItem("1002", 1, 5.0);
// 输出购物车中的商品信息
System.out.println("购物车中的商品信息:");
Map<String, Integer> items = cart.getCartItems();
for (String productId : items.keySet()) {
System.out.println("商品编号:" + productId
+ ",数量:" + items.get(productId));
}
// 更新购物车中商品数量
cart.update("1001", 3, 10.0);
// 输出购物车中的商品信息
System.out.println("购物车中的商品信息:");
items = cart.getCartItems();
for (String productId : items.keySet()) {
System.out.println("商品编号:" + productId
+ ",数量:" + items.get(productId));
}
// 删除购物车中的商品
cart.removeItem("1002", 5.0);
// 输出购物车中的商品信息
System.out.println("购物车中的商品信息:");
items = cart.getCartItems();
for (String productId : items.keySet()) {
System.out.println("商品编号:" + productId
+ ",数量:" + items.get(productId));
}
// 清空购物车
cart.clear();
// 输出购物车中的商品数量和总价格
System.out.println("购物车中商品数量:" + cart.getItemCount());
System.out.println("购物车中商品总价钱:" + cart.getTotalPrice());
}
}
watch: { '$store.state.crossborderCartList': { handler: function(newVal, oldVal) { this.count = 0; newVal.forEach(data => { data.cartItems.forEach(item => { this.count += item.quantity; }); }); }, deep: true } },让页面一进入就加载计算
这段代码是一个 Vue 组件中的 watch 属性,用于监听 Vuex store 中的 crossborderCartList 对象的变化。一旦 crossborderCartList 对象发生变化,就会触发该函数中的代码,重新计算 count 的值。
如果想要页面一进入就加载计算,可以将该函数提取出来,然后在组件的 created 生命周期钩子函数中调用一次。代码如下:
```
watch: {
'$store.state.crossborderCartList': {
handler: 'updateCount',
deep: true
}
},
created() {
this.updateCount(this.$store.state.crossborderCartList);
},
methods: {
updateCount(newVal, oldVal) {
this.count = 0;
newVal.forEach(data => {
data.cartItems.forEach(item => {
this.count += item.quantity;
});
});
}
}
```
阅读全文
相关推荐
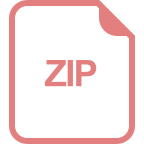
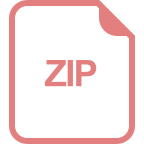
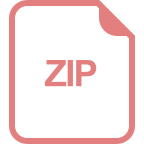
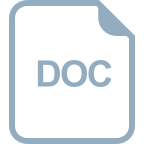












