如何将vector<string>中的值遍历
时间: 2024-05-08 14:18:32 浏览: 147
您可以使用循环来遍历vector<string>中的值,如下所示:
```
vector<string> strVec = {"hello", "world", "!"};
for (int i = 0; i < strVec.size(); i++) {
cout << strVec[i] << endl;
}
```
输出结果为:
```
hello
world
!
```
您也可以使用C++11引入的新循环方式来遍历vector<string>中的值:
```
vector<string> strVec = {"hello", "world", "!"};
for (const auto& str : strVec) {
cout << str << endl;
}
```
输出结果为:
```
hello
world
!
```
相关问题
vector<vector<string>>怎么遍历
你可以使用两个嵌套的循环来遍历 `vector<vector<string>>`。第一个循环用于遍历外层的 `vector`,第二个循环用于遍历内层的 `vector`。下面是一个示例代码:
```cpp
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::vector<std::string>> matrix = {
{"a", "b", "c"},
{"d", "e", "f"},
{"g", "h", "i"}
};
for (const auto& row : matrix) {
for (const auto& element : row) {
std::cout << element << " ";
}
std::cout << std::endl;
}
return 0;
}
```
以上代码将遍历并打印出 `matrix` 中的所有元素。你可以根据你的需求修改内部循环中的代码来执行你想要的操作。
vector<string>遍历
可以使用for循环或者迭代器进行遍历。例如:
```
vector<string> vec = {"hello", "world", "how", "are", "you"};
for(int i = 0; i < vec.size(); i++){
cout << vec[i] << " ";
}
// 或者使用迭代器
vector<string>::iterator it;
for(it = vec.begin(); it != vec.end(); it++){
cout << *it << " ";
}
```
希望这个回答对您有所帮助。
阅读全文
相关推荐
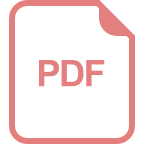
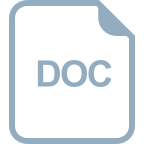
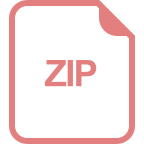













