state = torch.FloatTensor(state.reshape(1, -1)).to(self.device)
时间: 2024-06-05 20:07:59 浏览: 16
这段代码是将输入的状态 state 转换为 PyTorch 中的张量(tensor)格式,并通过 .to(self.device) 将其放到指定的设备上进行计算,例如 GPU 或 CPU。
更具体地说,torch.FloatTensor() 将输入的 state 转换为浮点型的张量,而 reshape(1, -1) 则将 state 转换为一个 1 行 N 列的矩阵,其中 N 是 state 的维度。这样做是为了将单个状态转换为一个 batch,方便在模型中进行处理。最后, .to(self.device) 方法将张量放到指定的设备上,self.device 是在模型初始化时指定的设备,通常是 GPU。
相关问题
我有两个文件run.py 和model.py 其中model.py如下所示: class EGAE(torch.nn.Module): def clustering(self): epsilon = torch.tensor(10**-7).to(self.device) indicator = self.indicator / self.indicator.norm(dim=1).reshape((self.data_size, -1)).max(epsilon) indicator = indicator.detach().cpu().numpy() km = KMeans(n_clusters=self.n_clusters).fit(indicator) prediction = km.predict(indicator) acc, nmi, ari, f1 = cal_clustering_metric(self.labels.cpu().numpy(), prediction) return acc, nmi, ari, f1 现在我要在run.py文件中调用model.py中EGAE类里面的clustering方法,并且输出clustering方法中prediction
可以按照以下步骤在run.py中调用model.py中EGAE类的clustering方法,并输出prediction:
1. 导入需要的库和模块:
```python
import torch
from model import EGAE
from sklearn.cluster import KMeans
```
2. 加载已经训练好的模型和数据:
```python
# 加载数据
data = ...
labels = ...
# 加载模型
model = EGAE(...)
model.load_state_dict(torch.load("model.pth"))
model.eval()
```
3. 调用EGAE类中的clustering方法,并输出prediction:
```python
# 调用clustering方法
with torch.no_grad():
acc, nmi, ari, f1, prediction = model.clustering(data, labels)
# 输出prediction
print(prediction)
```
其中,需要注意以下几点:
- 在调用EGAE类中的clustering方法时,需要传入data和labels作为参数;
- 在调用模型之前,需要先加载训练好的权重,并将模型设置为eval模式;
- 在调用clustering方法时,需要使用with torch.no_grad()语句块来禁用梯度计算,以提高推理速度;
- EGAE类中的clustering方法需要返回acc、nmi、ari、f1和prediction这五个值,需要将其全部返回并接收;
def forward(self, x, state=None, flow=None, mode='bilinear'): # pylint: disable=unused-argument, arguments-differ # Check size assert len(x.size()) == 5, 'Input tensor must be BxTxCxHxW.' b, timesteps, c, h, w = x.size() assert c == self.input_size, f'feature sizes must match, got input {c} for layer with size {self.input_size}' # recurrent layers rnn_output = [] rnn_state = torch.zeros(b, self.hidden_size, h, w, device=x.device) if state is None else state for t in range(timesteps): x_t = x[:, t] if flow is not None: rnn_state = warp_features(rnn_state, flow[:, t], mode=mode) # propagate rnn state rnn_state = self.gru_cell(x_t, rnn_state) rnn_output.append(rnn_state) # reshape rnn output to batch tensor return torch.stack(rnn_output, dim=1)这段代码的计算过程
这段代码实现了一个带有 GRU 循环神经网络的前向传播过程。输入张量 x 的维度为 BxTxCxHxW,其中 B 是批大小,T 是时间步数,C 是通道数,H 和 W 分别是张量的高度和宽度。该函数首先会检查输入张量的维度是否正确。
接下来,函数会初始化 GRU 循环神经网络的状态 rnn_state。如果输入参数 state 不为空,则将其作为初始状态;否则,将状态初始化为全零张量。
接着,函数会遍历时间步,每次取出输入张量 x 中的一个时间步 x_t。如果输入参数 flow 不为空,则使用 warp_features 函数对 rnn_state 进行变形,变形的方式由参数 mode 决定。
然后,函数将 x_t 和 rnn_state 作为 GRU 循环神经网络的输入,计算得到 rnn_state。将 rnn_state 添加到 rnn_output 列表中。
最后,函数将 rnn_output 列表中的张量沿着时间步维度进行堆叠,得到输出张量。输出张量的维度为 BxTxCxHxW。
相关推荐
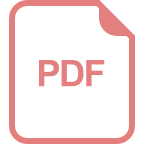
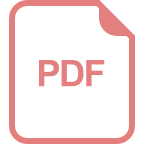
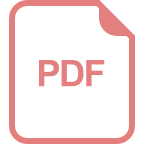












