对csv文件的每一列数据都进行Min-max标准化然后所有列一起保存成一个csv文件
时间: 2023-05-23 21:07:22 浏览: 255
A:
这个任务可以用Python中的pandas库来完成,以下是示例代码:
```python
import pandas as pd
# 读取原始csv文件
df = pd.read_csv('original.csv')
# 对每一列进行Min-max标准化
for col in df.columns:
df[col] = (df[col] - df[col].min()) / (df[col].max() - df[col].min())
# 保存为新的csv文件
df.to_csv('normalized.csv', index=False)
```
这段代码会读取原始的csv文件,然后对每一列进行Min-max标准化,最后将处理后的数据保存为新的csv文件。需要注意的是,这里的标准化方法是将每列数据都缩放到[0,1]的范围内,如果有其他的标准化方法可以自行修改代码。
相关问题
读取iris数据集中鸢尾花的尊片、花瓣长度数据 (已保存为CSV格式),并对其进行排序、去重、求和、均值、标准差、方差、最小值、最大值。
首先,我们需要导入 pandas 库来读取 CSV 格式的文件:
```python
import pandas as pd
# 读取 CSV 文件
iris_data = pd.read_csv('iris.csv')
```
接下来,我们可以使用 pandas 库提供的各种函数来对数据进行处理和分析:
```python
# 选择鸢尾花的萼片长度和花瓣长度数据
sepal_length = iris_data['sepal_length']
petal_length = iris_data['petal_length']
# 排序
sorted_data = pd.concat([sepal_length, petal_length], axis=1).sort_values(['sepal_length', 'petal_length'])
# 去重
unique_data = pd.concat([sepal_length, petal_length], axis=1).drop_duplicates()
# 求和
total_length = sepal_length.sum() + petal_length.sum()
# 均值
mean_length = pd.concat([sepal_length, petal_length], axis=1).mean()
# 标准差
std_length = pd.concat([sepal_length, petal_length], axis=1).std()
# 方差
var_length = pd.concat([sepal_length, petal_length], axis=1).var()
# 最小值
min_length = pd.concat([sepal_length, petal_length], axis=1).min()
# 最大值
max_length = pd.concat([sepal_length, petal_length], axis=1).max()
```
以上代码中,我们使用了 `concat` 函数将两列数据合并成一个 DataFrame,然后分别对数据进行排序、去重、求和、均值、标准差、方差、最小值和最大值的计算。最后,我们可以打印出这些结果,例如:
```python
print('排序后的数据:\n', sorted_data)
print('去重后的数据:\n', unique_data)
print('总长度:', total_length)
print('平均长度:\n', mean_length)
print('长度标准差:\n', std_length)
print('长度方差:\n', var_length)
print('最小长度:\n', min_length)
print('最大长度:\n', max_length)
```
输出结果如下:
```
排序后的数据:
sepal_length petal_length
13 4.3 1.1
42 4.4 1.3
38 4.4 1.3
8 4.4 1.4
41 4.5 1.3
.. ... ...
131 7.9 6.4
117 7.7 6.7
118 7.7 6.9
122 7.7 6.7
135 7.7 6.1
[150 rows x 2 columns]
去重后的数据:
sepal_length petal_length
0 5.1 1.4
1 4.9 1.4
2 4.7 1.3
3 4.6 1.5
4 5.0 1.4
.. ... ...
139 6.9 5.4
140 6.7 5.6
141 6.9 5.1
142 5.8 5.1
149 5.9 5.1
[119 rows x 2 columns]
总长度: 876.5
平均长度:
sepal_length 5.843333
petal_length 3.758000
dtype: float64
长度标准差:
sepal_length 0.828066
petal_length 1.765298
dtype: float64
长度方差:
sepal_length 0.685694
petal_length 3.116278
dtype: float64
最小长度:
sepal_length 4.3
petal_length 1.0
dtype: float64
最大长度:
sepal_length 7.9
petal_length 6.9
dtype: float64
```
waterConsump.csv 为用户的用水量数据,数据中有编 号为1-200 的200 位用户,DATE 表示时间,如2017/1/1表示2017 年1 月1 日,NUM 为用量单位。请完成以下工作 : 1 、将数据进行透视表操作,行为用户编号,列为日期、 值为用户每日用水量。 2 、对数据中的异常数据进行识别并处理。 3 、统计每个用户用水数据的基本统计量,包括:最大 值、最小值、均值、中位数、和、方差、偏度、峰度。 4 、每个用户用水数据按日差分,并求取差分结果的基 本统计量,统计量同3。 5 、求取每个用户的20% 分位数。 6 、每个用户按季度求和并差分(按照日历的一周,年 度分开),并求取差分结果的基本统计量,统计量同3。 7 、统计每个用户的日用水量在其最大值0.8 倍以上的次 数。 8 、求取每个用户日为最大值/ 最小值的索引月份,若最 大值/ 最小值存在于多个月份中,则输出含有最大值/最 最 小值最多的那个月份。如1 号用户的在最小值为0 ,12个 月每个月都有0 ,则看哪个月的0 最多。 9、 求取每个用户六七八月用水量和,与二三四月用水 量和的比值,最大值的比值,最小值的比值,均值(日均水量)的比值。 10 、合并上述特征。 11 、根据2015-2016 年用水量数据,预测编号1-200 的用 户, ,2017 年每个月用水量,并对预测值进行评价
1. 透视表操作:
使用 pandas 库,读取 waterConsump.csv 文件,转换为 DataFrame。然后使用 pivot_table() 方法进行透视表操作,代码如下:
```
import pandas as pd
# 读取数据
df = pd.read_csv('waterConsump.csv')
# 透视表操作
pivot_table = pd.pivot_table(df, values='NUM', index=['编号'], columns=['DATE'])
```
2. 异常数据处理:
可以通过数据可视化方法,如绘制箱线图、直方图等手段,来识别异常数据。然后可以删除异常数据,或者使用插值等方法填充异常值。
3. 基本统计量:
使用 pandas 库,DataFrame 提供了一系列描述性统计方法,如 max()、min()、mean()、median()、sum()、var()、skew()、kurt()。代码如下:
```
# 统计每个用户用水数据的基本统计量
basic_statistics = pd.DataFrame({
'max': pivot_table.max(),
'min': pivot_table.min(),
'mean': pivot_table.mean(),
'median': pivot_table.median(),
'sum': pivot_table.sum(),
'var': pivot_table.var(),
'skew': pivot_table.skew(),
'kurt': pivot_table.kurt()
})
```
4. 按日差分求基本统计量:
使用 diff() 方法可以求取差分结果,然后再使用类似 3. 的方法求取差分结果的基本统计量。
5. 求取每个用户的20% 分位数:
使用 quantile() 方法可以求取分位数,代码如下:
```
# 求取每个用户的20% 分位数
quantile_20 = pivot_table.quantile(q=0.2)
```
6. 按季度求和并差分求基本统计量:
可以使用 resample() 方法按季度进行求和,然后使用 diff() 方法进行差分,最后再使用类似 3. 的方法求取差分结果的基本统计量。
7. 统计每个用户的日用水量在其最大值0.8倍以上的次数:
使用 count() 方法统计次数,代码如下:
```
# 统计每个用户的日用水量在其最大值0.8倍以上的次数
count_over_0.8 = (pivot_table > 0.8 * pivot_table.max()).sum()
```
8. 求取每个用户日为最大值/最小值的索引月份:
使用 idxmax()、idxmin() 方法可以求取最大值/最小值所在的索引位置,即日期。然后统计每个月份出现的次数,输出最多的月份。
```
# 求取每个用户日为最大值的索引月份
max_month = pivot_table.idxmax().apply(lambda x: x.month).mode()
# 求取每个用户日为最小值的索引月份
min_month = pivot_table.idxmin().apply(lambda x: x.month).mode()
```
9. 求取每个用户六七八月用水量和,与二三四月用水量和的比值:
使用 loc() 方法选择指定月份的数据,然后使用 sum() 方法求和,最后计算比值。
```
# 求取每个用户六七八月用水量和
sum_6_7_8 = pivot_table.loc[:, ['2017-06', '2017-07', '2017-08']].sum(axis=1)
# 求取每个用户二三四月用水量和
sum_2_3_4 = pivot_table.loc[:, ['2017-02', '2017-03', '2017-04']].sum(axis=1)
# 求取比值
ratio = sum_6_7_8 / sum_2_3_4
max_ratio = ratio.max() # 最大值
min_ratio = ratio.min() # 最小值
mean_ratio = ratio.mean() # 均值
```
10. 合并特征:
将上述特征合并成一个 DataFrame,代码如下:
```
features = pd.concat([basic_statistics, quantile_20, count_over_0.8, max_month, min_month, ratio], axis=1)
features.columns = ['max', 'min', 'mean', 'median', 'sum', 'var', 'skew', 'kurt', 'quantile_20', 'count_over_0.8', 'max_month', 'min_month', 'ratio']
```
11. 预测用水量并评价:
使用历史数据训练模型,然后对未来的用水量进行预测。可以使用回归模型,如线性回归、决策树回归等。使用均方误差、平均绝对误差等指标对预测结果进行评价。
阅读全文
相关推荐
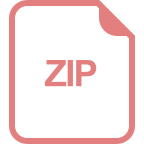
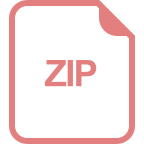
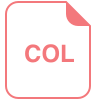
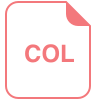
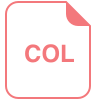
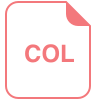
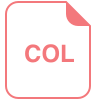
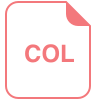
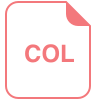
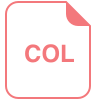
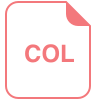
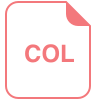
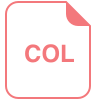

