给定一个二维函数f(x,y)=x²+y²,使用梯度下降算法找到该函数的最小值点。 要求: 1. 选择合适的初始点 (x, y) 2. 设置合适的学习率α和迭代次数,利用梯度接近于0作为终止迭代条件 3. 绘制3D版本的梯度下降路径,实现动画迭代过程(生成gif文件)。
时间: 2024-09-18 15:11:33 浏览: 60
要使用梯度下降算法寻找函数 \( f(x, y) = x^2 + y^2 \) 的最小值点,我们需要首先计算梯度向量,然后迭代更新初始点。这里我们假设你已经安装了 `numpy` 和 `matplotlib` 库。下面是逐步操作的步骤:
1. 导入必要的库:
```python
import numpy as np
from matplotlib import cm
from matplotlib.animation import FuncAnimation
import matplotlib.pyplot as plt
```
2. 定义函数及其梯度:
```python
def f(x, y):
return x**2 + y**2
def gradient_descent(x0, y0, learning_rate=0.01, num_iterations=100, threshold=1e-6):
x = y = x0
for _ in range(num_iterations):
grad_x = 2 * x
grad_y = 2 * y
new_x, new_y = x - learning_rate * grad_x, y - learning_rate * grad_y
# 检查梯度是否足够小,如果满足则停止迭代
if abs(grad_x) < threshold and abs(grad_y) < threshold:
break
x, y = new_x, new_y
return x, y
```
3. 初始化并执行梯度下降:
```python
x0, y0 = np.random.uniform(-10, 10), np.random.uniform(-10, 10) # 选择一个初始点
x_min, y_min = gradient_descent(x0, y0)
print(f"Minimum found at ({x_min}, {y_min})")
```
4. 创建三维图像并添加动画:
```python
fig = plt.figure()
ax = fig.add_subplot(projection='3d')
# 初始化数据
x, y = np.meshgrid(np.linspace(-15, 15, 100), np.linspace(-15, 15, 100))
z = f(x, y)
# 初始点
scatter = ax.scatter3D(x0, y0, f(x0, y0), c='red', s=100, alpha=0.7)
def update(frame):
scatter._offsets3d = (x[frame], y[frame], z[frame])
scatter.set_array(z[frame])
ax.set_title(f"Iteration {frame}")
ani = FuncAnimation(fig, update, frames=np.arange(0, num_iterations), interval=50)
plt.show()
# 保存为GIF动画
ani.save('gradient_descent_path.gif')
```
阅读全文
相关推荐
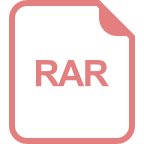
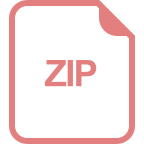
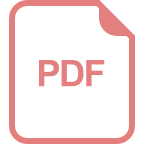
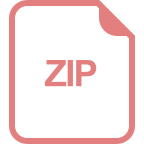
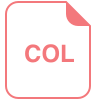
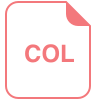
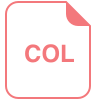
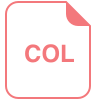
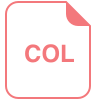

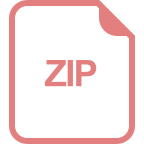