c语言使用BF算法进行匹配,并输出主串内容、子串内容以及在主串中的匹配位置。
时间: 2024-02-06 20:11:49 浏览: 72
好的,以下是使用C语言实现BF算法进行字符串匹配的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int BF_match(char *main_str, char *sub_str) {
int m = strlen(main_str);
int n = strlen(sub_str);
for (int i = 0; i <= m - n; i++) {
int j = 0;
while (j < n && main_str[i+j] == sub_str[j]) {
j++;
}
if (j == n) {
printf("主串内容:%s\n", main_str);
printf("子串内容:%s\n", sub_str);
printf("匹配位置:%d\n", i);
return i;
}
}
printf("在主串中未找到匹配的子串\n");
return -1;
}
int main() {
char main_str[] = "hello world";
char sub_str[] = "world";
BF_match(main_str, sub_str);
return 0;
}
```
以上代码中,`main_str`表示主串,`sub_str`表示要匹配的子串。函数返回子串在主串中的起始位置,如果未找到匹配的子串则返回-1。下面是使用示例:
```c
int main() {
char main_str[] = "hello world";
char sub_str[] = "world";
BF_match(main_str, sub_str);
return 0;
}
```
输出结果为:
```
主串内容:hello world
子串内容:world
匹配位置:6
```
希望这个例子可以帮助你理解BF算法的基本原理和C语言的实现方式。
阅读全文
相关推荐
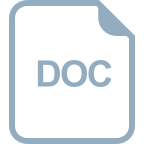
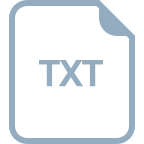















