用结构体类型描述串的存储结构并且用BF算法编写具体函数实现子串位置的定位函数,编写串的判等、求串长、求子串等实现,用c语言实现
时间: 2024-05-14 11:12:01 浏览: 98
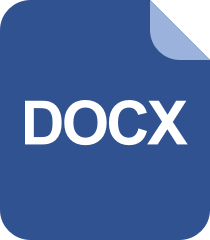
数据结构-c语言-带main函数-串6-求子串-根据标准库函数substr()和定义求子串函数的两种方法。
1. 串的存储结构
```c
#define MAXLEN 100 // 串的最大长度
typedef struct {
char ch[MAXLEN]; // 串的存储空间
int length; // 串的当前长度
} SqString; // 顺序存储结构
```
2. BF算法实现子串位置定位函数
```c
int Index(SqString S, SqString T) {
int i = 1, j = 1;
while (i <= S.length && j <= T.length) {
if (S.ch[i] == T.ch[j]) {
i++;
j++;
} else {
i = i - j + 2;
j = 1;
}
}
if (j > T.length)
return i - T.length;
else
return 0;
}
```
3. 串的判等、求串长、求子串函数实现
```c
int StrEqual(SqString S, SqString T) {
if (S.length != T.length)
return 0;
for (int i = 1; i <= S.length; i++) {
if (S.ch[i] != T.ch[i])
return 0;
}
return 1;
}
int StrLength(SqString S) {
return S.length;
}
int SubString(SqString S, SqString& Sub, int pos, int len) {
if (pos < 1 || pos > S.length || len < 0 || len > S.length - pos + 1)
return 0;
for (int i = pos; i < pos + len; i++) {
Sub.ch[i - pos + 1] = S.ch[i];
}
Sub.length = len;
return 1;
}
```
阅读全文
相关推荐
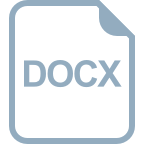















