写一个cpp程序,用bf算法在主串中匹配所有子串,并替换成另一种子串
时间: 2024-11-22 17:35:53 浏览: 0
BF(Boyer-Moore)算法是一种字符串搜索算法,用于快速查找主串中是否存在某个固定的子串。在C++中,我们可以创建一个函数来实现这个功能。这里是一个简单的示例:
```cpp
#include <iostream>
#include <string>
// Boyer-Moore 算法的核心部分
int bad_char_search(const std::string& text, const std::string& pattern) {
int n = text.length();
int m = pattern.length();
for (int i = m - 1; i < n; ) {
int j = m - 1;
while (j >= 0 && text[i] == pattern[j]) {
i--;
j--;
}
if (j < 0) { // 如果找到了,返回开始位置
return i + 1;
} else {
// 如果找不到,跳过模式中最后一个不匹配字符的位置
i += pattern[j + 1];
}
}
return -1; // 没有找到,返回 -1
}
// 替换子串的辅助函数
void replace_substring(std::string& main_str, const std::string& old_substr, const std::string& new_substr) {
size_t index = 0;
while ((index = main_str.find(old_substr, index)) != std::string::npos) {
main_str.replace(index, old_substr.size(), new_substr);
index += new_substr.size(); // 更新搜索起始位置
}
}
int main() {
std::string main_str = "Hello, World! This is a test string.";
std::string search_substr = "is";
std::string replacement_substr = "was";
// 使用 BF 算法找出子串的起始位置
for (size_t pos : {bad_char_search(main_str, search_substr), bad_char_search(main_str, replacement_substr)}) {
if (pos != -1) {
std::cout << "Found '" << search_substr << "' at position: " << pos << std::endl;
// 将找到的子串替换为新子串
replace_substring(main_str, search_substr, replacement_substr);
// 打印处理后的字符串
std::cout << "After replacing: " << main_str << std::endl;
}
}
return 0;
}
```
在这个程序中,我们首先定义了一个`bad_char_search`函数,用于应用BF算法寻找子串。然后,在`main`函数中,我们对两个子串(一个是待搜索的,另一个是替换成的新字符串)分别进行了搜索和替换。
阅读全文
相关推荐
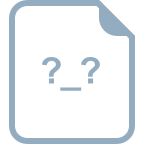
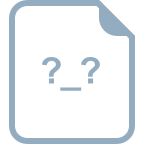
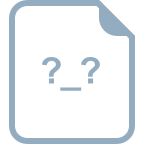








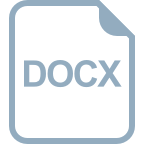
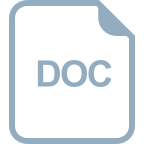
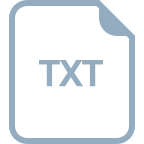
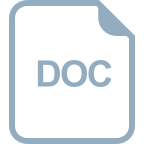
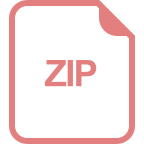
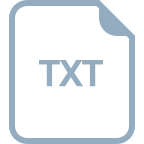
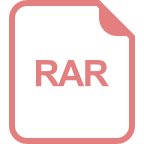