用bf算法再次实现上述操作
时间: 2024-10-28 22:10:24 浏览: 9
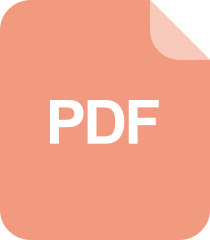
python通过BF算法实现关键词匹配的方法
使用Brute Force (BF) 算法,即朴素的模式匹配方法,可以在字符串中逐个检查所有可能的子串来查找匹配的模式。这种方法虽然简单直观,但是当主串和模式都非常长时,其时间复杂度将会非常高。以下是使用BF算法的基本实现:
```cpp
#include <iostream>
#include <string>
#include <vector>
// Brute Force 搜索函数
bool bruteForceSearch(const std::string& text, const std::string& pattern) {
size_t text_len = text.length();
size_t pattern_len = pattern.length();
for (size_t i = 0; i <= text_len - pattern_len; ++i) {
bool match = true;
for (size_t j = 0; j < pattern_len; ++j) {
if (text[i + j] != pattern[j]) {
match = false;
break;
}
}
if (match) {
std::cout << "Pattern found at index " << i << "\n";
return true;
}
}
return false;
}
int main() {
std::string text = "ABABCABABC";
std::string pattern = "ABC";
if (bruteForceSearch(text, pattern)) {
std::cout << "Pattern found.\n";
} else {
std::cout << "Pattern not found.\n";
}
return 0;
}
```
在这个例子中,我们遍历主串`text`的所有子串,每次比较长度为`pattern_len`的子串是否等于`pattern`。如果找到匹配,则打印出该子串的起始位置。
然而,注意BF算法在这种情况下不是最佳选择,因为它的时间复杂度是O(n*m),其中n是主串长度,m是模式长度,对于大型数据集来说效率非常低。因此,对于实际应用,通常会选择KMP或其他更为高效的字符串匹配算法。
阅读全文
相关推荐
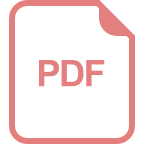
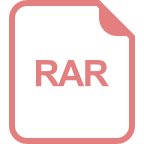

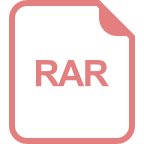
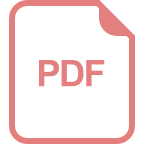
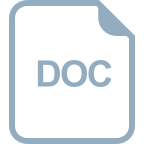
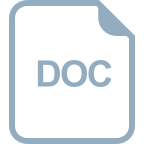
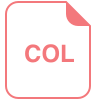
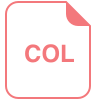
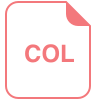
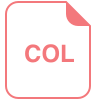
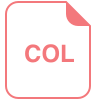
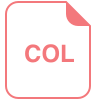
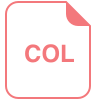
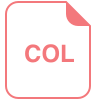
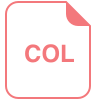
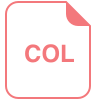
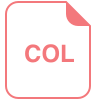