Python缓存机制:优化算法性能的秘密武器详解
发布时间: 2024-08-31 13:34:49 阅读量: 106 订阅数: 47 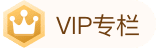
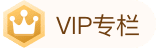
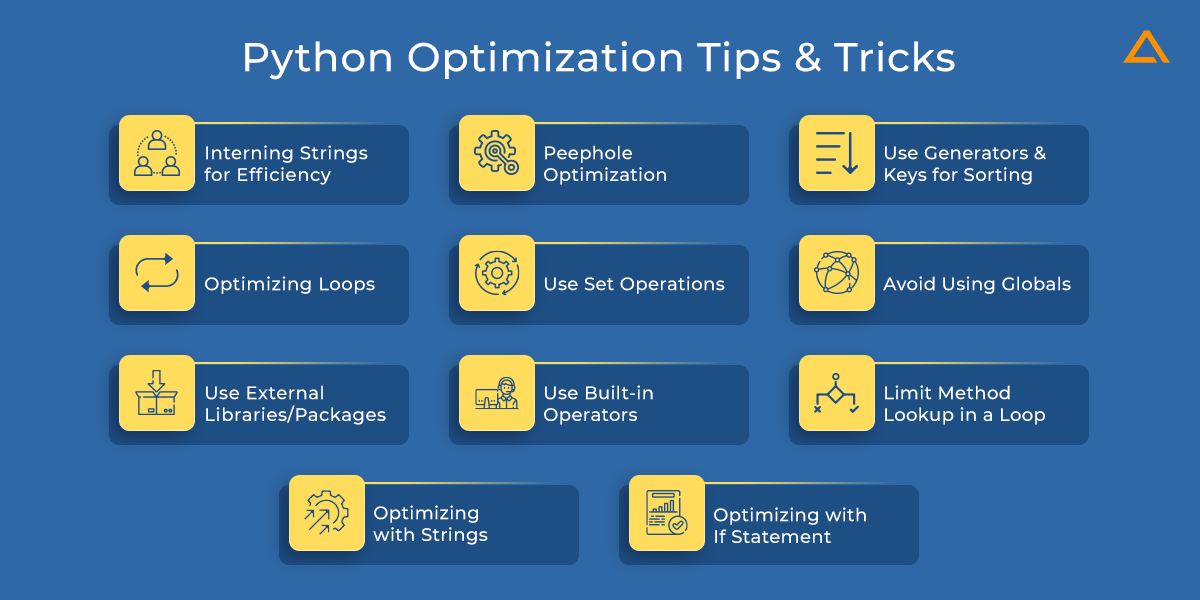
# 1. Python缓存机制概述
缓存机制是现代计算机科学中用于提升数据访问效率的一种技术手段。在Python中,合理的缓存应用不仅能减少不必要的计算,还可以极大地优化算法性能。缓存通过存储频繁使用的数据来减少数据检索的时间,从而加速数据访问,尤其在需要处理大量数据或执行复杂计算的场合,使用缓存机制可以显著提升性能和响应速度。
缓存技术在多种应用场景中扮演着关键角色,从简单的函数结果缓存到复杂的分布式系统中的数据缓存,都有着广泛的应用。Python的内置库如`functools`和`weakref`提供了基本的缓存工具,使得开发者能够方便地在应用中实施缓存策略。
在接下来的章节中,我们将深入探讨Python缓存技术的基础知识,包括其基本原理、内置缓存机制、不同的缓存策略与算法,以及这些技术如何应用到实际的问题中去优化性能。
# 2. Python缓存技术基础
## 2.1 缓存的基本原理
### 2.1.1 缓存的定义和作用
缓存是一种存储临时数据的技术,旨在减少数据访问时间和提高系统的性能。它的工作原理是将频繁使用的数据或计算结果存储在快速访问的硬件或软件层面上,比如RAM内存或硬盘上的缓存区。在计算机系统中,缓存可以显著减少访问主存或磁盘的需要,因为这些操作通常比从缓存中读取数据要慢得多。
缓存的作用可概述为以下几点:
- **加速数据访问:** 通过缓存减少数据检索时间,提高数据处理速度。
- **减少数据重复计算:** 将计算结果暂存于缓存,避免重复计算。
- **降低系统负载:** 减少对后端存储系统的压力,使得系统更稳定。
### 2.1.2 缓存与算法性能的关系
缓存与算法性能之间有着密切的联系。当算法访问数据时,如果该数据已经被缓存,那么访问速度会大大加快。特别是在处理大数据集时,合理利用缓存可以显著提高算法的效率。
好的缓存策略能够:
- **提高命中率:** 即提高从缓存中获取数据的概率,降低访问慢速存储的次数。
- **降低延迟:** 减少数据访问的等待时间。
- **提升吞吐量:** 提高系统单位时间内处理请求的能力。
## 2.2 Python内置缓存机制
### 2.2.1 函数缓存:functools.lru_cache
Python的`functools`模块提供了`lru_cache`装饰器,实现了最近最少使用(LRU)算法的缓存机制。通过简单地装饰一个函数,就可以缓存这个函数的返回值,当相同的参数再次被传递给函数时,可以直接从缓存中返回结果,而不是重新计算。
以下是一个使用`lru_cache`的示例代码:
```python
from functools import lru_cache
@lru_cache(maxsize=128)
def fib(n):
if n < 2:
return n
return fib(n-1) + fib(n-2)
# 第一次调用fib(10)将进行计算,之后调用时将直接返回结果
print(fib(10)) # 输出结果
```
参数说明:
- `maxsize`:缓存的最大容量。超出这个容量时,最早加入的缓存将被清除。
代码逻辑分析:
- `@lru_cache`装饰器用于缓存函数`fib`的结果。
- 当`fib`函数被调用时,`lru_cache`首先检查参数是否已经被缓存。
- 如果缓存存在,直接返回缓存的结果;如果不存在,计算结果后将其缓存。
### 2.2.2 对象缓存:weakref.WeakKeyDictionary
`weakref.WeakKeyDictionary`是Python提供的另一种缓存机制,它允许存储对象作为键的字典,且这些键对象不会阻止键对象被垃圾回收器回收。这种方式适用于当键对象不再被使用时,自动清理缓存。
以下是一个使用`WeakKeyDictionary`的示例代码:
```python
from weakref import WeakKeyDictionary
class Example:
def __init__(self, value):
self.value = value
def __repr__(self):
return f"Example({self.value})"
cache = WeakKeyDictionary()
key = Example(10)
cache[key] = 'cached value'
print(cache[key]) # 输出:cached value
del key
# 由于key没有被其他引用,它将被垃圾回收
# WeakKeyDictionary会自动清理对应的缓存项
print(cache) # 输出:{},缓存已被清理
```
## 2.3 缓存策略与算法
### 2.3.1 最近最少使用(LRU)算法
LRU(Least Recently Used)算法是一种常用的缓存策略,它的核心思想是“最近被访问过的数据在将来被访问的概率更高”。在LRU缓存中,当缓存满了时,最先被添加进缓存的数据会被删除,也就是最长时间未被访问的数据。
LRU算法的实现可以通过链表或双向链表来完成,每个节点代表一个缓存项,最近使用的节点移动到链表头部,最不常用的节点保留在尾部。当需要删除节点时,直接删除尾部的节点即可。
### 2.3.2 先进先出(FIFO)与最近最常用(LFU)算法
FIFO(First In First Out)是最早进入缓存的数据将最早被移除的策略,而LFU(Least Frequently Used)则是最不经常被使用的数据将被移除。FIFO的实现简单,只需要一个队列即可,但可能不会考虑到数据的局部性原理。LFU则可以更有效地处理局部性原理,但实现复杂度较高。
FIFO算法的Python实现可以用队列来模拟,而LFU则需要维护一个计数器来记录每个缓存项的访问频率,并在缓存满时移除频率最低的项。
现在,我们已经探索了Python缓存技术的基础。在下一章,我们将深入探讨缓存机制在算法优化中的具体应用。
# 3. 缓存机制在算法优化中的应用
随着信息技术的不断进步,软件系统面临的数据量和计算需求日益增长。为了提高算法性能,缓存机制已经成为不可或缺的技术之一。缓存能够显著减少数据访问时间,提升算法效率,尤其在数据结构、网络编程和大数据处理等领域中应用广泛。
## 3.1 缓存策略在数据结构中的应用
缓存策略在数据结构中的应用主要体现在对频繁访问数据的快速读取上。缓存可以被看作是一种
0
0
相关推荐
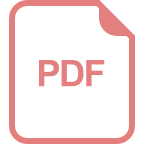
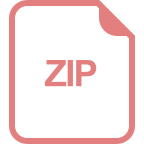
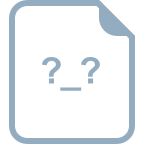





