Python分治算法优化:高效处理问题的艺术
发布时间: 2024-08-31 14:07:37 阅读量: 169 订阅数: 71 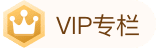
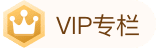
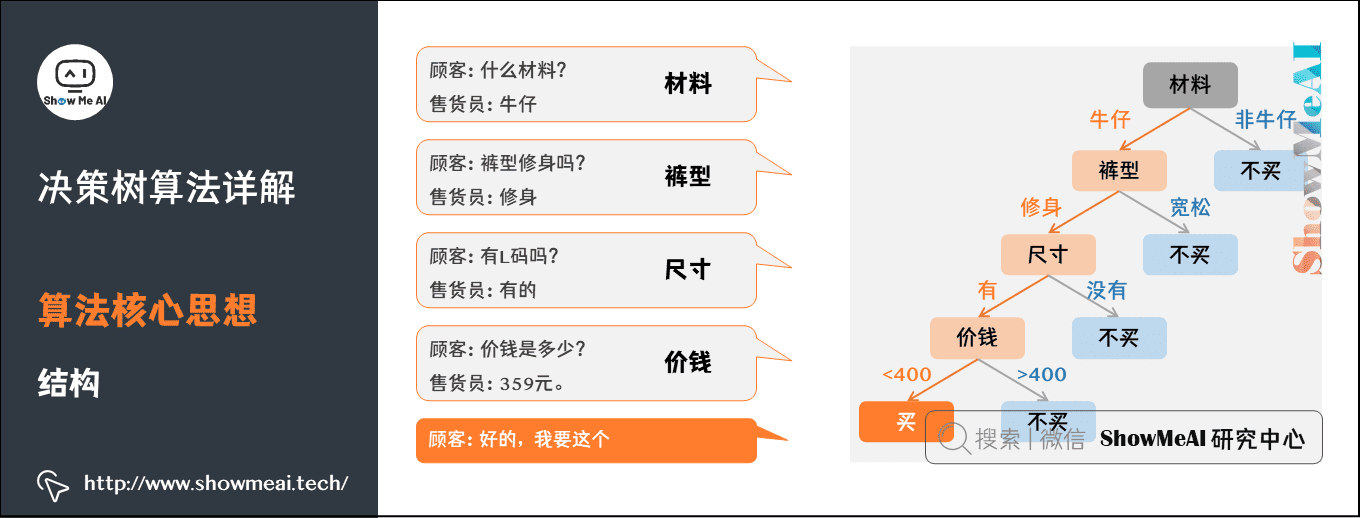
# 1. 分治算法的理论基础与Python实现
在本章节中,我们首先介绍分治算法的基本概念和理论基础。分治算法的核心思想是“分而治之”,即将复杂的问题分解为规模较小的相同问题,递归求解这些子问题,然后将子问题的解合并以得到原问题的解。我们将详细解释分治算法的工作原理,通过递归流程图展示其解决问题的步骤,并通过数学逻辑阐述为什么分治方法能够有效地简化问题。
随后,我们将以Python语言为例,展示如何实现分治算法。通过具体的代码示例,我们将演示分治策略在实际编程中的应用。这一部分将包括算法的Python伪代码实现,以及关键步骤的解释和代码注释。
以下是Python实现的分治算法的一个简单示例代码:
```python
def divide_and_conquer проблемы, 参数):
"""
分治算法的Python实现。
参数:
问题 -- 待解决的问题。
参数 -- 算法运行所需的相关参数。
返回:
解决方案 -- 问题的解决方案。
"""
if 问题足够简单:
return 直接解决问题
else:
sub_problems = 划分问题(问题)
solutions = [divide_and_conquer(sub_problem, 参数) for sub_problem in sub_problems]
return 合并解决方案(solutions)
```
在这个示例中,我们使用伪代码来描述分治算法的流程。请注意,这是一个通用的框架,具体的实现将依赖于要解决的问题类型和子问题的划分方式。
# 2. 分治算法在实际问题中的应用
分治算法作为一种有效的解决问题的策略,不仅仅在理论上有其独特的魅力,它在实际问题中的应用更是广泛而深远。本章将深入探讨分治算法如何应用于不同领域的实际问题,并展示其解决问题的强大能力。
### 2.1 分治算法在计算机科学中的应用
计算机科学领域是分治算法应用最为广泛的领域之一。从排序算法到复杂数据结构的构建,再到大规模数据处理,分治算法都扮演着核心角色。
#### 排序算法:归并排序
归并排序是分治算法在排序问题中的一个典型应用。通过递归将一个大数组分割成两个小数组,然后递归地排序这两个子数组,最后将排序好的子数组合并成一个有序数组。
```python
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2 # 找到中间位置,分割数组
L = arr[:mid]
R = arr[mid:]
merge_sort(L) # 递归排序左半部分
merge_sort(R) # 递归排序右半部分
i = j = k = 0
# 合并两个有序数组
while i < len(L) and j < len(R):
if L[i] < R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
# 检查是否还有元素未合并
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
return arr
# 使用示例
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(sorted_arr)
```
在这段代码中,我们首先将数组分为两半,然后递归地对每一半进行排序,最后通过合并操作得到最终的有序数组。归并排序的时间复杂度为O(n log n),在很多情况下都是一个非常有效的方法。
#### 数据结构:二分搜索树
在数据结构的构建中,分治算法也被广泛应用。例如,在构建二分搜索树(BST)的过程中,我们可以递归地选择一个元素作为根节点,然后将剩余元素分为两个子集,一个比该元素小,另一个比它大,分别递归构建左右子树。
```python
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(root, value):
if root is None:
return TreeNode(value)
if value < root.value:
root.left = insert(root.left, value)
elif value > root.value:
root.right = insert(root.right, value)
return root
# 使用示例
root = TreeNode(20)
insert(root, 15)
insert(root, 25)
insert(root, 12)
insert(root, 18)
```
这段代码展示了如何递归地构建一个二分搜索树。通过比较节点值,递归地将新值插入到树中正确的位置。
### 2.2 分治算法在工程问题中的应用
除了计算机科学,分治算法在工程、物理、经济等领域也有着广泛的应用。在这些领域中,分治算法通常用于解决大规模、复杂的优化问题。
#### 工程设计:电路设计的优化
在电路设计领域,分治算法可以用来优化电路布局,减少信号传输的延迟。通过将大的电路板分割成较小的部分,分别优化每一部分,然后再将它们组合起来,可以提高整体电路的工作效率。
#### 物理模拟:流体动力学分析
流体动力学的模拟问题往往具有复杂的数据依赖和巨大的计算量。分治算法可以帮助我们将流体域分割成小的子域,对每个子域进行模拟,然后通过接口条件来同步各个子域的结果,最终合成整个系统的解。
### 2.3 分治算法在日常生活中的应用
分治策略同样存在于我们的日常生活当中,它可以协助我们更有效地解决问题,即使这些问题看起来和计算机或科学技术毫无关系。
#### 生活决策:复杂项目管理
在生活中,我们可能需要管理一个复杂的项目,如婚礼策划或房屋装修。通过将项目分解为多个小任务,我们可以更容易地跟踪进度,分配资源,并在必要时进行调整,从而提高管理效率。
### 结语
分治算法通过将大问题分解为小问题,并独立解决每个小问题,然后将解合并以得到原始问题的解决方案,已成为处理各种类型问题的有效工具。从计算机科学到工程问题,再到日常生活中的应用,分治算法的存在证明了其普适性和强大能力。本章的介绍仅为冰山一角,而实际应用的深度与广度远超于此。随着问题的复杂性增加,分治策略将继续在各个领域发挥其独特而关键的作用。
通过本章的介绍,我们已经了解到分治算法在实际问题中的多种应用,并展示了如何将这一理论应用到不同的场景中。下一章我们将深入了解如何对分治算法进行优化,使其更加高效地解决实际问题。
# 3. Python中的分治算法优化策略
## 3.1 算法优化的基本理论
在探讨分治算法的优化策略之前,必须先理解算法优化的基本理论。算法优化是指在有限的资源约束下,改进算法的性能指标,如时间复杂度、空间复杂度以及代码的可读性和可维护性。优化过程分为两个主要方向:算法层面的优化和实现层面的优化。
### 算法层面优化
算法层面的优化通常涉及对问题本质的深入理解,通过数学工具和理论分析来改进算法。对于分治算法而言,这可能包括:
- **减少递归深度**:通过调整递归方程,减少算法需要执行的递归调用的次数。
- **合并步骤优化**:在递归的合并步骤中寻找更高效的方法来组合子问题的解。
- **减少冗余计算**:识别并消除算法中不必要的重复计算。
### 实现层面优化
实现层面的优化关注于代码层面的性能提升,这可能涉及到:
- **避免不必要的数据结构复制**:在合并步骤中,通过共享数据结构而不是复制它们来节省时间和空间。
- **利用缓存**:通过局部性原理,合理使用内存缓存,以减少对主存的访问次数。
- **并行计算**:在多核处理器中,通过并行化算法的某些部分来加速计算。
## 3.2 针对Python语言的优化技术
Python作为一种解释型语言,由于其动态类型和全局解释器锁(GIL)的特性,在性能上相比编译型语言有一定劣势。然而,通过以下优化技术可以最大限度地提升Python中分治算法的性能。
### 利用局部性原理
在Python中,对列表和字典等数据结构的操作具有局部性原理。优化技术之一是将常用的元素放在列表或字典的前面,这样在迭代和查找时就可以减少搜索时间和空间。
### 并行计算
Python的`multiprocessing`库可以让我们在多个CPU核心上运行代码。通过将分治算法的不同部分放在不同的进程中并行处理,可以显著提高性能。
```python
from multiprocessing import Pool
def divide_conquer_problem(subproblem):
# 分治算法的子问题求解函数
pass
def parallel_divide_conquer(problems):
with Pool() as pool:
results = pool.map(divide_conquer_problem, problems)
return results
#
```
0
0
相关推荐
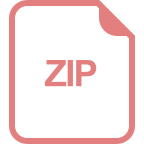
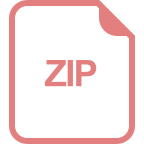
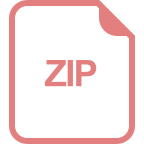
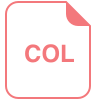
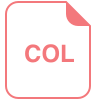
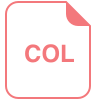
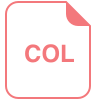
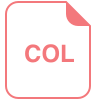
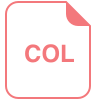