Python函数式编程技巧:优化算法代码的6种方法
发布时间: 2024-08-31 13:47:12 阅读量: 389 订阅数: 47 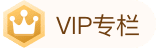
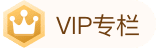
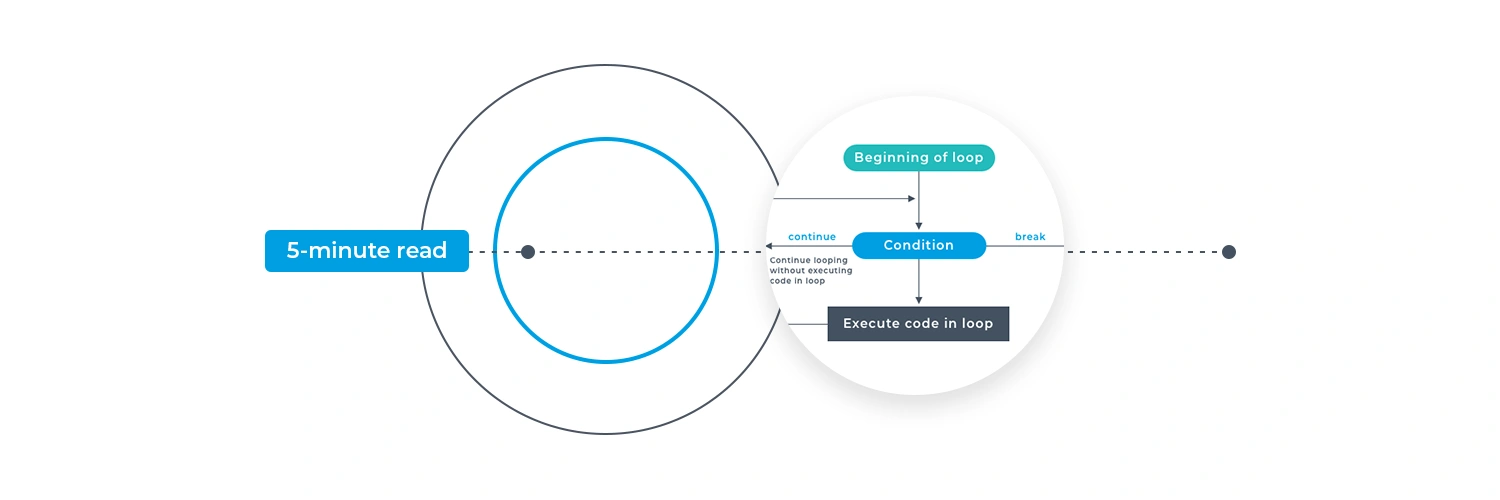
# 1. Python函数式编程基础
## 1.1 函数式编程简介
函数式编程(Functional Programming)是一种编程范式,它将计算视作数学函数的评估,并避免改变状态和可变数据。在Python中,函数式编程可以通过内置的高阶函数、匿名函数(lambda表达式)、以及列表解析等实现。这种编程范式强调不可变性和纯函数,这使得代码更加清晰,易于测试和维护。
## 1.2 函数式编程的优势
函数式编程的优势在于其简洁性和表达能力。通过使用函数式编程,可以减少代码的副作用,这有助于创建更加稳定和可预测的软件。此外,由于函数式编程中的函数通常是第一类对象(first-class objects),它们可以作为参数传递给其他函数,作为其他函数的返回值,或者被赋值给变量。
```python
# 示例:使用匿名函数和map函数进行计算
square = lambda x: x * x
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(square, numbers))
print(squared_numbers)
# 输出: [1, 4, 9, 16, 25]
```
通过以上简单的例子可以看出,Python通过简单直观的方式支持函数式编程特性。本章将深入探讨如何利用这些特性解决实际编程问题。
# 2. 高级函数式编程技术
## 2.1 不可变数据结构的使用
不可变数据结构是函数式编程的核心概念之一。不可变性意味着一旦数据结构被创建,它的内容就不能被更改。这与传统的可变数据结构形成鲜明对比,后者允许在程序运行时更改数据结构的内容。不可变数据结构为编程提供了一种安全性和可预测性,这是函数式编程的一大优势。
### 2.1.1 不可变数据结构的定义与好处
不可变数据结构的定义非常直接:它们是不能被修改的数据结构。在Python中,这通常意味着创建了一个对象后,你无法更改该对象的值,而是创建并返回一个新的对象以反映任何更改。
使用不可变数据结构的好处包括:
- **线程安全**: 不可变对象可以被多个线程安全地共享,因为它们不会被改变,所以不存在数据竞争的问题。
- **易于推理**: 不可变对象的状态是静态的,这使得理解和推理程序的行为变得更加容易。
- **防止副作用**: 在函数式编程中,我们鼓励编写没有副作用的函数。不可变数据结构自然支持这一理念,因为它们不能被修改。
- **持久性数据结构**: 不可变数据结构可以高效地复用旧数据结构的一部分,这是构建持久性数据结构的基础。
### 2.1.2 常见的不可变数据类型实例
在Python中,尽管没有原生的不可变数据结构类型,但某些类型在实践中被当做不可变类型来使用。这些类型包括:
- **字符串**: 一旦创建,字符串的内容就不能更改。
- **元组**: 元组中的元素一旦创建后不可更改。
- **frozenset**: 这是一种不可变且无序的集合类型,元素不能被修改。
```python
# 示例:使用元组不可变性
a = (1, 2, 3)
# a[0] = 10 # 这将会抛出TypeError,因为元组是不可变的
# 创建一个新元组来表示修改后的数据
b = (10,) + a[1:]
```
在上面的例子中,我们尝试修改元组`a`的第一个元素,结果会抛出一个`TypeError`异常,因为元组是不可变的。取而代之的是,我们创建了一个包含所需新值的新元组`b`。
## 2.2 闭包和装饰器
闭包和装饰器是函数式编程中两个重要的概念,它们增强了Python语言的功能性。
### 2.2.1 闭包的概念与应用
闭包是由函数及其相关的引用环境组合而成的一种实体。简单来说,闭包允许一个函数访问并操作函数外部的变量。
闭包的主要特点包括:
- **外部函数返回内部函数**: 闭包通常涉及到一个外部函数和一个内部函数。
- **内部函数引用外部函数的变量**: 内部函数会保存外部函数中定义的局部变量的引用。
- **外部函数的变量作用域**: 外部函数中定义的局部变量保持在内存中,即使外部函数已经执行完毕。
闭包的应用:
- **数据隐藏和封装**: 闭包可以封装私有状态,对外部代码隐藏这些状态。
- **创建工厂函数**: 闭包可以在运行时创建函数,这些函数根据创建它们的环境有着不同的行为。
```python
def multiplier_of(n):
def multiplier(number):
return number * n
return multiplier
double = multiplier_of(2)
triple = multiplier_of(3)
print(double(5)) # 输出: 10
print(triple(5)) # 输出: 15
```
在上述示例中,`multiplier_of`是一个工厂函数,它返回了一个闭包`multiplier`。每个闭包都保留了`multiplier_of`中`n`的值。
### 2.2.2 装饰器的原理与用法
装饰器是用一个函数来增强另一个函数的行为的函数。本质上,装饰器是一个闭包,它接受一个函数作为参数,并返回一个新的函数。
装饰器的原理:
- 装饰器是一个函数,它接受一个函数对象`f`作为参数。
- 它返回一个新的函数,这个新函数通常会在调用原始函数之前或之后执行一些操作。
- 新函数通常具有相同的名称和参数列表,以便于替换原始函数。
装饰器的用法:
- **日志记录**: 装饰器可以用来记录函数的调用日志。
- **性能分析**: 装饰器可以用来分析函数执行的时间。
- **权限检查**: 在函数执行前检查用户权限。
- **缓存**: 如果函数结果可以被缓存,可以使用装饰器来优化性能。
```python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
```
在以上示例中,`my_decorator`是一个装饰器,它在`say_hello`函数前后添加了额外的操作。
## 2.3 高阶函数的运用
高阶函数是那些可以接受其他函数作为参数或返回其他函数作为结果的函数。这种类型的函数在函数式编程中非常强大,它们增加了编程语言的表达能力。
### 2.3.1 高阶函数简介
高阶函数通常用于:
- **抽象**: 可以编写通用代码来操作其他函数,从而抽象出更通用的概念。
- **组合**: 将多个函数组合在一起,创建一个执行更复杂任务的新函数。
- **模块化**: 把程序分解成更小的、可重用的部分。
Python内置的一些高阶函数包括:
- `map()`
- `filter()`
- `reduce()`
### 2.3.2 map、filter、reduce函数详解
这三个函数是高阶函数的典型例子,分别用于映射、过滤和累积操作。
- **map(function, iterable, ...)**: 对`iterable`中每个项应用`function`,并返回一个迭代器。
```python
numbers = [1, 2, 3, 4, 5]
squared = map(lambda x: x * x, numbers)
print(list(squared)) # 输出: [1, 4, 9, 16, 25]
```
- **filter(function, iterable)**: 过滤`iterable`,只保留`function`返回`True`的项。
```python
numbers = [1, 2, 3, 4, 5]
even = filter(lambda x: x % 2 == 0, numbers)
print(list(even)) # 输出: [2, 4]
```
- **reduce(function, iterable[, initializer])**: 对`iterable`应用`function`,将`function`应用于前两个元素并迭代。
```python
from functools import reduce
numbers = [1, 2, 3, 4, 5]
summed = reduce(lambda x, y: x + y, numbers)
print(summed) # 输出: 15
```
通过这些函数,我们可以用非常简洁和表达性强的方式编写代码,它们的组合使用可以实现很多复杂的操作。
# 3. 函数式编程实践技巧
在第二章中,我们已经深入探讨了函数式编程的核心概念,包括高级函数的运用和高阶函数的运用。现在让我们深入实践,了解如何将函数式编程技
0
0
相关推荐
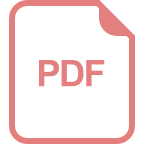
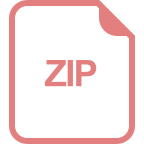
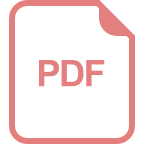





