(2) 若子串会在主串中出现多次,代码设计实现模式匹配BF算法支持多次匹配。c++
时间: 2024-10-14 14:12:36 浏览: 52
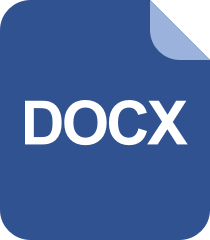
数据结构C++ 串的基本操作和BF算法与KMP算法的比较—适合初学者和复习.docx

Bollinger Bands (布林带) 是一种技术分析工具,用于衡量股票价格的波动范围,通常结合移动平均线一起使用。在C++中实现支持多次匹配的Brute Force (暴力搜索) 算法,如查找子串在一个字符串中出现的所有位置,可以采用以下步骤:
```cpp
#include <iostream>
#include <string>
// 函数用于在主串中找到子串的所有实例
std::vector<int> findPattern(const std::string& mainStr, const std::string& pattern) {
int n = mainStr.length();
int m = pattern.length();
std::vector<int> occurrences;
// 使用两个指针 i 和 j
for (int i = 0; i <= n - m; ++i) {
bool isMatch = true;
for (int j = 0; j < m; ++j) {
if (mainStr[i + j] != pattern[j]) {
isMatch = false;
break;
}
}
if (isMatch) {
occurrences.push_back(i);
}
}
return occurrences;
}
int main() {
std::string mainStr = "Hello, Hello, World!";
std::string pattern = "Hello";
std::vector<int> matches = findPattern(mainStr, pattern);
if (matches.empty()) {
std::cout << "Pattern not found in the main string.\n";
} else {
std::cout << "Pattern found at indices: ";
for (int index : matches) {
std::cout << index << ", ";
}
std::cout << "\n";
}
return 0;
}
```
在这个例子中,`findPattern` 函数通过迭代主串的每个字符位置,检查当前子串是否与给定的模式匹配。如果找到匹配,就将起始位置添加到结果向量中。在`main`函数中,我们调用这个函数并打印出所有匹配的位置。
阅读全文
相关推荐
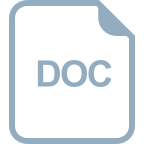
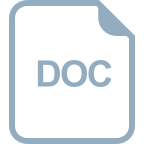
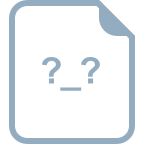
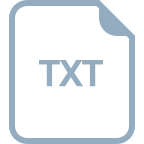
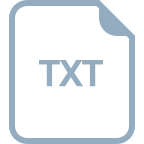
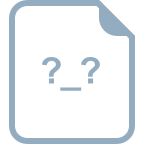
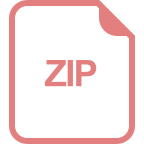
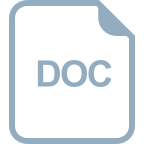
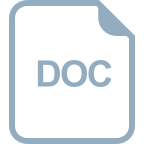
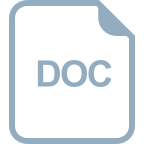







