假设串采用顺序串存储结构。设计一个算法求串t在串s中出现的次数,如果不是子串返回0。基于BF算法求解。例如"aa"在"aaaab"中出现2次。用C语言编写
时间: 2024-10-11 21:10:10 浏览: 48
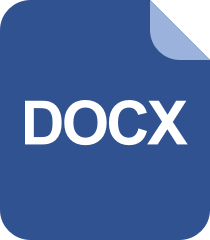
求串中最长重复子串。
为了使用广度优先搜索(BFS,即朴素的Brute Force Search)算法在一个字符串 `s` 中查找另一个字符串 `t` 的出现次数,你可以创建一个简单的遍历过程。这里是一个基本的 C 语言实现示例:
```c
#include <stdio.h>
#include <stdbool.h>
#include <string.h>
// 函数声明
int stringSearch(char *s, char *t);
int main() {
char s[] = "aaaab";
char t[] = "aa";
int count = stringSearch(s, t);
printf("'%s' 在 '%s' 中出现了 %d 次\n", t, s, count);
return 0;
}
// 定义字符串搜索函数
int stringSearch(char *s, char *t) {
int si = 0; // s 的指针
int ti = 0; // t 的指针
int count = 0;
while (si < strlen(s)) {
if (ti == strlen(t)) { // 如果 t 已经遍历完
count++;
ti = 0; // 从头开始检查下一个位置
} else if (s[si] != t[ti]) { // 如果当前字符不匹配
si++; // 移动到下个字符
ti = 0; // 重置 t 的指针
} else { // 匹配
si++, ti++; // 同时移动 s 和 t 的指针
}
}
return count; // 返回 t 在 s 中的出现次数
}
```
这个算法会逐个比较 `s` 中的每个子串是否与 `t` 相同。如果找到匹配,则继续寻找下一个;如果不匹配则跳过并检查下一个可能的位置。当 `t` 遍历完毕但在 `s` 中还有剩余字符时,表示 `t` 还有一次出现。
阅读全文
相关推荐
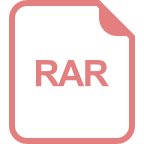
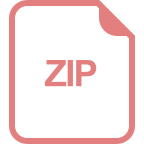















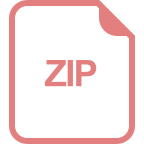