std::string 实现删除字符串中指定字符
时间: 2024-05-07 11:20:35 浏览: 14
可以使用 `std::string::erase()` 函数删除字符串中指定字符。该函数的参数是指定要删除的字符的位置和数量。可以使用 `std::string::find()` 函数在字符串中查找指定字符的位置,然后使用 `std::string::erase()` 函数删除该字符。以下是一个示例代码:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "hello world";
char ch = 'l';
std::size_t pos = str.find(ch);
while (pos != std::string::npos) {
str.erase(pos, 1);
pos = str.find(ch);
}
std::cout << str << std::endl;
return 0;
}
```
在上面的示例代码中,首先定义了一个字符串 `str` 和一个要删除的字符 `ch`。然后使用 `std::string::find()` 函数查找 `ch` 在 `str` 中的位置,并使用 `std::string::erase()` 函数删除该字符。由于 `std::string::find()` 函数返回的是 `std::string::npos`,表示未找到指定字符,因此在一个循环中执行删除操作,直到找不到指定字符为止。最后输出删除指定字符后的字符串。
相关问题
std::string 实现删除字符串中首尾空白
下面是一个实现删除字符串中首尾空白的示例代码:
```
#include <iostream>
#include <string>
std::string trim(const std::string& str) {
size_t start = str.find_first_not_of(" \t\n\r");
size_t end = str.find_last_not_of(" \t\n\r");
if (start == std::string::npos || end == std::string::npos) {
return "";
}
return str.substr(start, end - start + 1);
}
int main() {
std::string str = " \t Hello, World! \n\r ";
std::cout << "[" << str << "]" << std::endl;
std::cout << "[" << trim(str) << "]" << std::endl;
return 0;
}
```
在上面的代码中,我们定义了一个 `trim` 函数,它接受一个 `std::string` 类型的参数 `str`,并返回一个新的字符串,该字符串是 `str` 去除首尾空白后的结果。
在 `trim` 函数中,我们首先使用 `find_first_not_of` 函数来查找 `str` 中第一个不是空白字符的位置,然后使用 `find_last_not_of` 函数来查找 `str` 中最后一个不是空白字符的位置。如果找不到这样的位置,说明字符串全部都是空白字符,直接返回空字符串。
最后,我们使用 `substr` 函数从 `str` 中截取去除首尾空白后的子串,并返回该子串作为结果。
std::string移除指定字符串
你可以使用标准库中的字符串操作函数来移除指定的字符串。下面是一个示例代码,展示如何使用std::string的erase和find函数来移除指定字符串:
```cpp
#include <iostream>
#include <string>
void removeSubstring(std::string& mainStr, const std::string& subStr) {
// 查找子字符串
size_t pos = mainStr.find(subStr);
if (pos != std::string::npos) {
// 删除子字符串
mainStr.erase(pos, subStr.length());
}
}
int main() {
std::string str = "Hello World!";
std::string subStr = "o";
removeSubstring(str, subStr);
std::cout << "Modified string: " << str << std::endl;
return 0;
}
```
在上面的示例中,定义了一个`removeSubstring`函数,它接受一个`std::string`的引用和一个要移除的子字符串。该函数首先使用`find`函数查找子字符串在主字符串中的位置,并将结果保存在`pos`变量中。如果找到了子字符串,则使用`erase`函数从主字符串中删除该子字符串。
在主函数中,我们使用示例字符串"Hello World!"和要移除的子字符串"o"进行测试。运行后,输出结果为"Modified string: Hell Wrld!",子字符串"o"已被成功移除。
希望这个示例能够帮助到你!如果有更多问题,请随时提问。
相关推荐
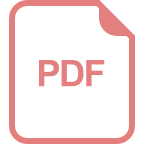
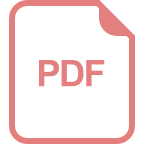
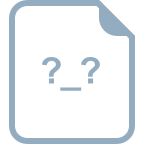
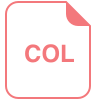
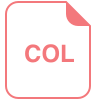
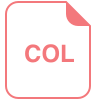
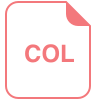
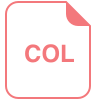







