std::stringstream 取出字符串
时间: 2023-12-14 16:03:22 浏览: 37
可以使用 `std::stringstream` 的 `str()` 方法来获取字符串流中的字符串。
以下是一个示例:
```c++
#include <iostream>
#include <string>
#include <sstream>
int main() {
std::stringstream ss;
ss << "Hello, world!" << std::endl;
std::string str = ss.str();
std::cout << "The string is: " << str;
return 0;
}
```
输出:
```
The string is: Hello, world!
```
在这个示例中,我们首先创建了一个 `std::stringstream` 对象 `ss`,并使用 `<<` 运算符将一个字符串和一个换行符插入到字符串流中。然后,我们使用 `str()` 方法将字符串流中的内容提取出来,并将其存储在 `std::string` 对象 `str` 中。最后,我们将这个字符串打印到标准输出中。
相关问题
string将字符串根据空格分割
可以使用C++ STL中的stringstream来实现字符串的分割,具体代码如下:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
using namespace std;
int main() {
string str = "hello world welcome to cpp";
stringstream ss(str); // 将字符串转换成stringstream类型
vector<string> result; // 定义一个vector来存储分割后的字符串
string temp;
while (ss >> temp) { // 当stringstream还有值时,不断取出单词
result.push_back(temp); // 将单词存入vector中
}
for (auto s : result) { // 输出vector中的每个单词
cout << s << endl;
}
return 0;
}
```
运行结果:
```
hello
world
welcome
to
cpp
```
C++16进制转字符串
可以使用C++中的stringstream和iomanip库来实现16进制转字符串的操作。下面是一个示例代码:
```C++
#include <iostream>
#include <sstream>
#include <iomanip>
using namespace std;
int main() {
string hex_str = "48656c6c6f20576f726c6421"; // 16进制字符串
stringstream ss;
for (int i = 0; i < hex_str.length(); i += 2) {
string byte_str = hex_str.substr(i, 2); // 取出每个字节的字符串表示
char byte = (char) strtol(byte_str.c_str(), NULL, 16); // 将字符串转为整数
ss << byte; // 写入到stringstream对象中
}
string str = ss.str(); // 从stringstream对象中取出转换后的字符串
cout << str << endl; // 输出字符串
return 0;
}
```
输出结果为:
```
Hello World!
```
相关推荐
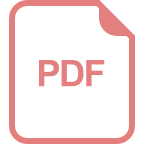
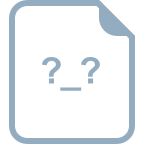











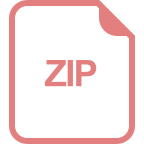
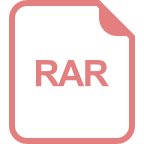