kotlin如何使用netty
时间: 2024-02-04 07:37:56 浏览: 27
在Kotlin中使用Netty,需要先引入Netty的依赖库,比如:
```
dependencies {
implementation 'io.netty:netty-all:4.1.53.Final'
}
```
然后可以按照Netty官方文档的示例实现自己的网络应用。例如,以下是一个简单的Echo服务器示例:
```kotlin
import io.netty.bootstrap.ServerBootstrap
import io.netty.buffer.ByteBuf
import io.netty.buffer.Unpooled
import io.netty.channel.ChannelHandlerContext
import io.netty.channel.ChannelInboundHandlerAdapter
import io.netty.channel.ChannelInitializer
import io.netty.channel.nio.NioEventLoopGroup
import io.netty.channel.socket.SocketChannel
import io.netty.channel.socket.nio.NioServerSocketChannel
class EchoServerHandler : ChannelInboundHandlerAdapter() {
override fun channelRead(ctx: ChannelHandlerContext, msg: Any) {
val buf = msg as ByteBuf
val data = ByteArray(buf.readableBytes())
buf.readBytes(data)
val response = Unpooled.copiedBuffer(data)
ctx.write(response)
}
override fun channelReadComplete(ctx: ChannelHandlerContext) {
ctx.flush()
}
override fun exceptionCaught(ctx: ChannelHandlerContext, cause: Throwable) {
cause.printStackTrace()
ctx.close()
}
}
fun main() {
val bossGroup = NioEventLoopGroup()
val workerGroup = NioEventLoopGroup()
try {
val bootstrap = ServerBootstrap()
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel::class.java)
.childHandler(object : ChannelInitializer<SocketChannel>() {
override fun initChannel(ch: SocketChannel) {
ch.pipeline().addLast(EchoServerHandler())
}
})
val future = bootstrap.bind(8080).sync()
future.channel().closeFuture().sync()
} finally {
workerGroup.shutdownGracefully()
bossGroup.shutdownGracefully()
}
}
```
在这个示例中,我们创建了一个Echo服务器,当客户端发送数据时,服务器会将数据原样返回给客户端。服务器使用了NIO的事件循环组(NioEventLoopGroup)来处理网络事件,同时使用了ServerBootstrap来启动服务器。在ChannelInitializer的初始化方法中,我们将EchoServerHandler添加到SocketChannel的处理器链中,用于处理接收到的数据。最后,我们绑定了8080端口并等待服务器关闭。
相关推荐
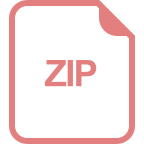
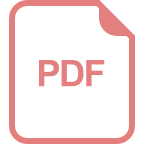
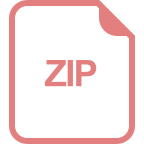














