C#中,将单通道的byte[]类型的图片数据保存为图片
时间: 2024-09-12 15:10:12 浏览: 52
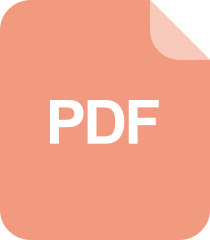
c#中实现图片灰度化技术详解
在C#中,如果你有一个单通道(灰度或索引颜色)的`byte[]`类型的图像数据,你可以使用`System.Drawing`命名空间下的`Bitmap`类来创建并保存这个图像。以下是一个简单的步骤:
1. 首先,你需要创建一个新的`Bitmap`实例,并指定宽度和高度,以及位深度(对于单通道,通常位深度是1(表示灰度)或4(索引颜色))。
```csharp
using System.Drawing;
...
int width = <图片宽度>;
int height = <图片高度>;
int pixelDepth = 1; // 灰度图像
Bitmap bitmap = new Bitmap(width, height, pixelDepth);
```
2. 接下来,使用`LockBits`方法获取像素数组,然后将你的`byte[]`数据复制到这个数组中。这里假设你的`byte[]`数据是从左上角开始存储的。
```csharp
byte[] imageData = ...; // 单通道图像数据
int bytesPerPixel = 1; // 对于单通道,每像素占用1字节
int offset = 0;
// Lock the bitmap's bits for read-write access
ImageAttributes attributes = new ImageAttributes();
attributes.SetColorKey(Color.Transparent, Color.Transparent);
using (Graphics g = Graphics.FromImage(bitmap))
{
IntPtr scan0 = bitmap.LockBits(
new Rectangle(0, 0, width, height),
ImageLockMode.ReadWrite,
PixelFormat.Format8bppIndexed
);
try
{
byte* ptr = (byte*)scan0.Scan0;
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++, offset++)
{
ptr[offset] = imageData[offset]; // 设置像素值
}
ptr += bytesPerPixel * width; // move to next line
}
}
finally
{
bitmap.UnlockBits(scan0);
}
}
```
3. 最后,你可以选择任意一种支持的图片格式,比如`.bmp`, `.jpg`, 或者`.png`,然后使用`Save`方法保存图片。
```csharp
string filePath = "<你的保存路径>";
bitmap.Save(filePath, ImageFormat.Bmp); // 例如,可以替换为其他格式
```
阅读全文
相关推荐
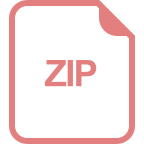
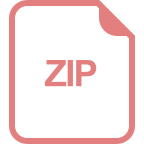
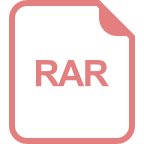
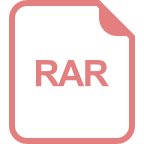
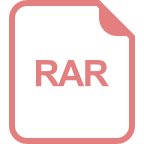
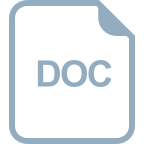
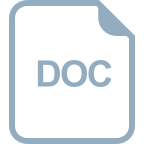
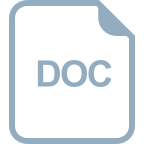
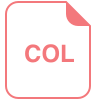
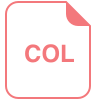
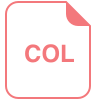





