给我提供一个java实现的httpUtils类,要求能发请求,且get请求带请求体
时间: 2024-03-03 10:51:01 浏览: 22
以下是一个简单的Java实现的HTTP工具类,支持发送GET、POST请求,以及GET请求带请求体:
```java
import java.io.*;
import java.net.*;
public class HttpUtils {
public static String sendGet(String url, String params) throws Exception {
String fullUrl = url;
if (params != null && !params.isEmpty()) {
fullUrl += "?" + params;
}
URL obj = new URL(fullUrl);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
con.setRequestProperty("User-Agent", "Mozilla/5.0");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
}
public static String sendPost(String url, String params) throws Exception {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("User-Agent", "Mozilla/5.0");
con.setRequestProperty("Accept-Language", "en-US,en;q=0.5");
con.setDoOutput(true);
if (params != null && !params.isEmpty()) {
OutputStreamWriter writer = new OutputStreamWriter(con.getOutputStream());
writer.write(params);
writer.flush();
writer.close();
}
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
}
public static String sendGetWithBody(String url, String params) throws Exception {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("User-Agent", "Mozilla/5.0");
con.setRequestProperty("Accept-Language", "en-US,en;q=0.5");
con.setDoOutput(true);
if (params != null && !params.isEmpty()) {
OutputStreamWriter writer = new OutputStreamWriter(con.getOutputStream());
writer.write(params);
writer.flush();
writer.close();
}
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
}
}
```
这个工具类包含了三个方法:sendGet、sendPost、sendGetWithBody,分别用于发送GET请求、POST请求,以及GET请求带请求体。其中,sendGet和sendPost方法与常见的HTTP工具类类似,而sendGetWithBody方法则是采用POST请求来模拟GET请求带请求体的情况。需要注意的是,在使用sendGetWithBody方法时,需要将请求方法设置为POST,并在请求体中传递参数。
相关推荐
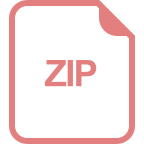














