java spring boot框架 如何运行sql脚本
时间: 2023-04-05 12:01:57 浏览: 91
您可以使用 Spring Boot 的 JdbcTemplate 来运行 SQL 脚本。首先,您需要在应用程序的配置文件中配置数据源。然后,您可以在应用程序中使用 JdbcTemplate 来执行 SQL 语句和脚本。例如,您可以使用以下代码来运行 SQL 脚本:
```
@Autowired
private JdbcTemplate jdbcTemplate;
public void runScript(String scriptPath) {
Resource resource = new ClassPathResource(scriptPath);
try {
String script = IOUtils.toString(resource.getInputStream(), StandardCharsets.UTF_8);
jdbcTemplate.execute(script);
} catch (IOException e) {
// handle exception
}
}
```
其中,`scriptPath` 是 SQL 脚本文件的路径,`jdbcTemplate` 是 Spring Boot 提供的 JDBC 模板。在 `runScript` 方法中,我们使用 `ClassPathResource` 来加载 SQL 脚本文件,然后使用 `jdbcTemplate.execute` 方法来执行 SQL 脚本。
相关问题
Spring Boot中自动执行sql脚本
使用Spring Boot可以自动执行SQL脚本,只需将SQL脚本文件放在特定的位置即可。以下是具体步骤:
1.创建一个名为“data.sql”的文件,并将其放在项目的根目录下。如果您使用的是其他文件名或位置,可以在application.properties文件中进行配置。
2.在data.sql文件中编写SQL查询,如下所示:
```
INSERT INTO users (user_name, password) VALUES ('user1', 'password1');
INSERT INTO users (user_name, password) VALUES ('user2', 'password2');
```
3.运行Spring Boot应用程序时,Spring Boot将自动执行data.sql文件中的SQL查询,并将数据插入数据库中。
请注意,如果您使用的是JPA或Hibernate等ORM框架,则应使用“import.sql”文件而不是“data.sql”文件,并将其放在类路径的根目录下。此外,还可以在application.properties文件中配置其他属性,例如:
```
spring.datasource.schema= # Schema (DDL) script resource reference.
spring.datasource.data=classpath:data.sql # Data (DML) script resource reference.
spring.datasource.initialize=true # Populate the database using 'data.sql' script.
spring.datasource.continue-on-error=false # Do not stop if an error occurs while initializing the database.
```
希望以上内容对您有所帮助!
在spring boot中,执行SQL脚本,往数据库中插入数据
可以使用Spring JDBC来执行SQL脚本并向数据库中插入数据。以下是一个简单的示例代码:
```java
@Autowired
JdbcTemplate jdbcTemplate;
public void executeSqlScript() throws IOException {
Resource resource = new ClassPathResource("data.sql");
InputStream inputStream = resource.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
String line;
while ((line = reader.readLine()) != null) {
jdbcTemplate.execute(line);
}
}
```
在这个示例中,我们使用JdbcTemplate来执行SQL脚本。我们通过Resource对象获取data.sql文件的InputStream,然后使用BufferedReader逐行读取脚本文件中的SQL语句。然后,我们使用JdbcTemplate的execute()方法来执行每个SQL语句。
请注意,这只是一个简单的示例,实际情况中可能需要更复杂的逻辑来处理错误和异常情况。
相关推荐
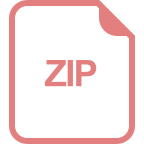
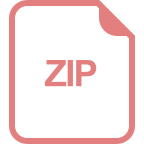












