def gcd(a, b): """求两个数的最大公约数""" while b: a, b = b, a % b return adef can_divide(s, n, m): """判断是否能平分可乐""" if s % gcd(n, m) == 0: return True else: return Falsedef min_pour_times(s, n, m): """计算最少要倒的次数""" if not can_divide(s, n, m): return "No" else: return gcd(s, gcd(n, m))
时间: 2024-03-29 18:35:52 浏览: 37
这段代码定义了三个函数:gcd、can_divide和min_pour_times。
gcd函数用于求两个数的最大公约数,采用的是辗转相除法。在while循环中,每次将b赋值给a,将a%b的结果赋值给b,直到b为0,此时a即为最大公约数,函数返回a。
can_divide函数用于判断可乐s能否平分成两个容量为n和m的瓶子中,采用的是判断s是否能被n和m的最大公约数整除的方式。若能整除,则返回True,否则返回False。
min_pour_times函数用于计算最少需要倒几次才能把容量为s的可乐平分到容量为n和m的两个瓶子中。首先调用can_divide函数判断是否能平分可乐,如果不能则返回"No",否则使用gcd函数分别计算s、n和m的最大公约数,并返回最终结果。
这三个函数可以用于解决关于瓶子和可乐的一些问题,例如上面提到的“计算最少需要倒几次才能把容量为s的可乐平分到容量为n和m的两个瓶子中”。
相关问题
def gcd(a, b): """ 求两个正数的最大公约数 """ while b: a, b = b, a % b return adef lcm(a, b): """ 求两个正数的最小公倍数 """ return a * b // gcd(a, b)if __name__ == '__main__': a = int(input("请输入第一个正数:")) b = int(input("请输入第二个正数:")) print("最大公约数为:", gcd(a, b)) print("最小公倍数为:", lcm(a, b))转化为C语言
下面是将 Python 代码转化为 C 语言代码的结果:
```
#include <stdio.h>
int gcd(int a, int b) {
while (b) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
int lcm(int a, int b) {
return a * b / gcd(a, b);
}
int main() {
int a, b;
printf("请输入第一个正数:");
scanf("%d", &a);
printf("请输入第二个正数:");
scanf("%d", &b);
printf("最大公约数为:%d\n", gcd(a, b));
printf("最小公倍数为:%d\n", lcm(a, b));
return 0;
}
```
需要注意的是,在 C 语言中,需要在使用变量前先声明。因此,我们需要在函数前面声明函数的名称和参数类型。此外,Python 中的多重赋值语句需要用中间变量来实现。
Adef vfunc(a,b): def vfunc(a, b): def vfunc(a, b): Ddef vfunc(a,b=2):
The first line contains a syntax error due to the missing colon at the end of the line. The correct syntax would be:
def vfunc(a, b):
The second line is a duplicate of the first line and should be removed.
The third line is also a duplicate of the first line and should be removed.
The fourth line is a valid function definition with a default value of 2 for the parameter b.
阅读全文
相关推荐
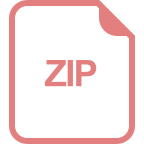
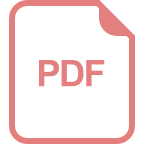
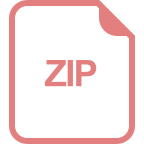
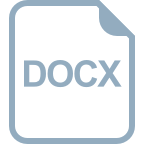
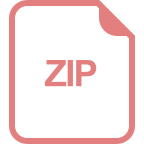
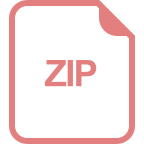
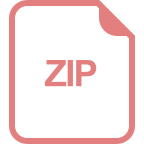
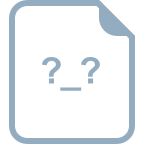
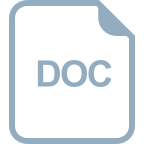
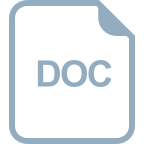
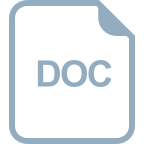


