stm32 esp8266怎么在ap模式下监听端口消息并发送给串口
时间: 2023-11-19 19:04:58 浏览: 38
要在ESP8266的AP模式下监听端口,并将接收到的消息发送给STM32的串口,你可以按照以下步骤进行操作:
1. 在ESP8266上创建一个AP,使其处于AP模式下。
2. 在ESP8266上开启TCP服务器,并在指定的端口处监听客户端连接。
3. 当客户端连接到ESP8266的TCP服务器时,ESP8266会接收到客户端发送的消息。
4. 在ESP8266上将接收到的消息发送给STM32的串口。
下面是一些代码示例,可以帮助你实现这个功能:
在ESP8266上创建AP和开启TCP服务器:
```python
import socket
# 设置ESP8266为AP模式
ap_ssid = "ESP8266-AP"
ap_password = "123456789"
ap_channel = 7
ap_hidden = False
ap_authmode = 3
ap_max_connection = 4
ap_ip_address = "192.168.4.1"
ap_subnet_mask = "255.255.255.0"
ap_gateway = "192.168.4.1"
ap_config = (ap_ssid, ap_password, ap_channel, ap_hidden, ap_authmode, ap_max_connection)
ap_if = network.WLAN(network.AP_IF)
ap_if.active(True)
ap_if.config(essid=ap_config[0], password=ap_config[1], channel=ap_config[2], hidden=ap_config[3], authmode=ap_config[4], max_connection=ap_config[5])
# 开启TCP服务器
tcp_server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
tcp_server.bind((ap_ip_address, 8080))
tcp_server.listen(1)
```
在ESP8266上监听客户端连接和接收消息:
```python
# 监听客户端连接
client, client_address = tcp_server.accept()
print("Client connected:", client_address)
# 接收消息并发送给STM32的串口
while True:
data = client.recv(1024)
if data:
print("Received data:", data)
uart.write(data)
```
在STM32上接收ESP8266发送的消息:
```c
// 初始化USART1串口
void usart1_init(void)
{
// 启用USART1时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
// 初始化GPIOA
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 将PA9和PA10引脚映射到USART1
GPIO_PinAFConfig(GPIOA, GPIO_PinSource9, GPIO_AF_1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource10, GPIO_AF_1);
// 初始化USART1
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 启用USART1
USART_Cmd(USART1, ENABLE);
}
// 从USART1接收数据
uint8_t usart1_receive(void)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART1);
}
// 向USART1发送数据
void usart1_send(uint8_t data)
{
USART_SendData(USART1, data);
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
}
```
通过以上步骤,你就可以在ESP8266的AP模式下监听端口消息,并将接收到的消息发送给STM32的串口了。
相关推荐
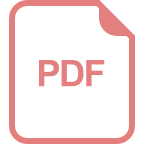
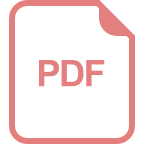
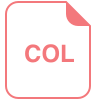
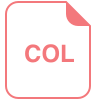
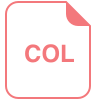
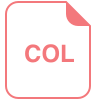
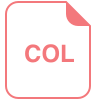









