管道通信通过c语言实现两个终端相互聊天
时间: 2023-10-11 15:05:38 浏览: 127
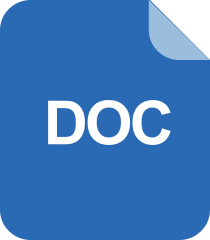
WINDOWS/C语言/管道通信实现机制

要通过 C 语言实现两个终端之间的相互聊天,可以使用命名管道(named pipe)来实现管道通信。以下是一个简单的实现:
1. 首先,需要创建两个命名管道,一个用于从终端1发送消息到终端2,另一个用于从终端2发送消息到终端1。可以使用`mkfifo`函数来创建命名管道:
```c
mkfifo("pipe1", 0666);
mkfifo("pipe2", 0666);
```
2. 然后,可以在两个进程中分别打开相应的管道,并执行读取和写入操作。例如,在终端1中可以运行以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd1 = open("pipe1", O_WRONLY); // 打开管道1,写入消息
int fd2 = open("pipe2", O_RDONLY); // 打开管道2,读取消息
char message[100];
while (1) {
printf("Enter message: ");
fgets(message, 100, stdin);
write(fd1, message, strlen(message) + 1); // 写入管道1
read(fd2, message, sizeof(message)); // 从管道2读取
printf("<terminal2>: %s", message);
}
close(fd1);
close(fd2);
return 0;
}
```
在终端2中可以运行以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd1 = open("pipe2", O_WRONLY); // 打开管道2,写入消息
int fd2 = open("pipe1", O_RDONLY); // 打开管道1,读取消息
char message[100];
while (1) {
printf("Enter message: ");
fgets(message, 100, stdin);
write(fd1, message, strlen(message) + 1); // 写入管道2
read(fd2, message, sizeof(message)); // 从管道1读取
printf("<terminal1>: %s", message);
}
close(fd1);
close(fd2);
return 0;
}
```
这些代码将从标准输入读取消息,并通过相应的管道发送消息。然后,从另一个管道中读取消息,并将其输出到标准输出。注意,在每个管道上,一个进程读取数据,另一个进程写入数据。
现在,你可以在两个终端上相互聊天了。
阅读全文
相关推荐


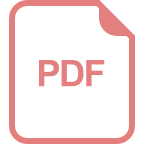
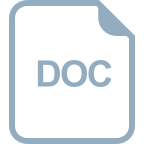
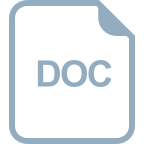
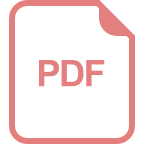
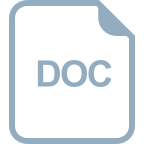
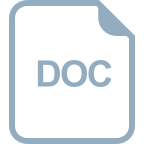
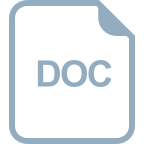
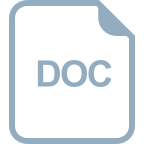
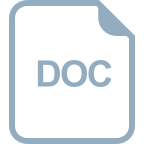
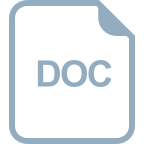
