可以写一段reactor的代码么
时间: 2024-05-12 18:19:02 浏览: 16
当然可以。以下是一个简单的Reactor代码示例,它使用了Java NIO库来实现异步非阻塞IO:
```
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
import java.util.Set;
public class Reactor implements Runnable {
private Selector selector;
private ServerSocketChannel serverSocket;
public Reactor(int port) throws IOException {
selector = Selector.open();
serverSocket = ServerSocketChannel.open();
serverSocket.socket().bind(new InetSocketAddress(port));
serverSocket.configureBlocking(false);
SelectionKey sk = serverSocket.register(selector, SelectionKey.OP_ACCEPT);
sk.attach(new Acceptor());
}
public void run() {
try {
while (!Thread.interrupted()) {
selector.select();
Set<SelectionKey> selected = selector.selectedKeys();
Iterator it = selected.iterator();
while (it.hasNext()) {
SelectionKey sk = (SelectionKey) it.next();
it.remove();
Runnable r = (Runnable) sk.attachment();
if (r != null) {
r.run();
}
}
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
class Acceptor implements Runnable {
public void run() {
try {
SocketChannel channel = serverSocket.accept();
if (channel != null) {
new Handler(selector, channel);
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
class Handler implements Runnable {
private ByteBuffer buffer = ByteBuffer.allocate(1024);
private SocketChannel channel;
public Handler(Selector selector, SocketChannel channel) throws IOException {
this.channel = channel;
channel.configureBlocking(false);
SelectionKey sk = channel.register(selector, SelectionKey.OP_READ);
sk.attach(this);
}
public void run() {
try {
int numBytes = channel.read(buffer);
if (numBytes == -1) {
channel.close();
return;
}
buffer.flip();
byte[] bytes = new byte[buffer.limit()];
buffer.get(bytes);
System.out.println("Received: " + new String(bytes));
buffer.clear();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
public static void main(String[] args) throws IOException {
Reactor reactor = new Reactor(8080);
new Thread(reactor).start();
}
}
```
该示例中的Reactor模式使用了Java NIO库中的Selector、ServerSocketChannel和SocketChannel等类。当有请求到达时,Acceptor类会接受连接,并注册一个新的Handler类以处理该连接。Handler类会读取请求,并将其打印到控制台。
该示例中的Reactor模式具有以下优点:
1. 可以处理多个连接,而不需要为每个连接创建一个线程。
2. 使用异步非阻塞IO,可以提高应用程序的性能和可伸缩性。
3. 可以通过添加新的Handler类来处理不同类型的请求,从而使应用程序更加灵活。
相关推荐
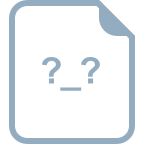











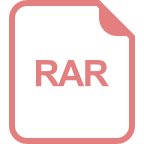
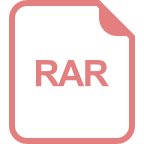