python给定一个字符串s和一个字符a,统计字符a在字符串s中出现的次数。字符范围为任意ascii字符,除了回车符
时间: 2024-03-12 22:48:51 浏览: 29
好的,可以使用Python的内置函数count()来计算字符a在字符串s中出现的次数。示例代码如下:
```python
s = "hello world"
a = "l"
count = s.count(a)
print("字符 {} 在字符串 {} 中出现的次数为:{}".format(a, s, count))
```
输出结果为:
```
字符 l 在字符串 hello world 中出现的次数为:3
```
需要注意的是,如果字符串s中存在回车符,则需要先将其替换掉再进行计数操作。可以使用replace()函数将回车符替换为空字符串,示例代码如下:
```python
s = "hello\nworld"
a = "l"
s = s.replace("\n", "")
count = s.count(a)
print("字符 {} 在字符串 {} 中出现的次数为:{}".format(a, s, count))
```
输出结果为:
```
字符 l 在字符串 helloworld 中出现的次数为:3
```
相关问题
给定一个字符串在字符串中找到一个连续出现至少k次的字符
可以使用滑动窗口的思想来解决这个问题。具体步骤如下:
1. 初始化滑动窗口的左右边界,以及一个长度为 26 的数组,用来记录每个字符出现的次数。
2. 遍历字符串,每遇到一个字符,就将该字符出现次数加 1。同时,将滑动窗口的右边界向右移动一位。
3. 如果当前滑动窗口中该字符出现的次数大于等于 k,就继续向右移动右边界,直到该字符出现次数小于 k。
4. 如果当前滑动窗口中所有字符都出现了至少 k 次,就更新最长连续子串的长度。
5. 如果当前滑动窗口中某个字符出现次数小于 k,就将该字符出现次数减 1,并将滑动窗口的左边界向右移动一位。
6. 重复上述步骤,直到遍历完整个字符串。
下面是示例代码实现:
```python
def find_longest_substring(s: str, k: int) -> int:
ans = 0
n = len(s)
freq = [0] * 26 # 记录每个字符出现的次数
# 滑动窗口的左右边界
left, right = 0, 0
while right < n:
# 将当前字符出现次数加 1
freq[ord(s[right]) - ord('a')] += 1
# 移动右边界
right += 1
# 如果当前滑动窗口中该字符出现的次数大于等于 k,就继续向右移动右边界,直到该字符出现次数小于 k
while left < right and min(freq) >= k:
# 更新最长连续子串的长度
ans = max(ans, right - left)
# 将左边界向右移动一位,并将对应字符出现次数减 1
freq[ord(s[left]) - ord('a')] -= 1
left += 1
return ans
```
注意,上述代码中使用了 ord() 函数将字符转换成 ASCII 码,从而方便地将字符转换成数字。
给定一个字符串,请用python表示出其中的#和*
### 回答1:
字符字符在字符在字字符在字母字符在字母表字符在字母表中字符在字母表中的字符在字母表中的索字符在字母表中的索引字符在字母表中的索引。
字符在字母表中的索引。
可以字符在字母表中的索引。
可以使用字符在字母表中的索引。
可以使用内字符在字母表中的索引。
可以使用内置字符在字母表中的索引。
可以使用内置函数字符在字母表中的索引。
可以使用内置函数ord字符在字母表中的索引。
可以使用内置函数ord()字符在字母表中的索引。
可以使用内置函数ord()和字符在字母表中的索引。
可以使用内置函数ord()和字符串字符在字母表中的索引。
可以使用内置函数ord()和字符串的字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s =字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = '字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c)字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) -字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a')字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') +字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1,字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end='字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
``字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
``字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
``字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其在字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其在字字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其在字母字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其在字母表字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其在字母表中字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其在字母表中的字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其在字母表中的索字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其在字母表中的索引字符在字母表中的索引。
可以使用内置函数ord()和字符串的遍历来实现。
例子:
```python
s = 'hello'
for c in s:
print(ord(c) - ord('a') + 1, end=' ')
```
输出:
```
8 5 12 12 15
```
解释:
字符串中第一个字符'h'在字母表中为第8个,第二个字符'e'为第5个,以此类推。因此,把每个字符转换成它在字母表中的对应数字即可。在这个例子中,我们对每个字符使用ord()函数获取其ASCII码值,然后减去'a'的ASCII码值,再加1即可得到其在字母表中的索引。
### 回答2:
回答:可以使用Python中的字符串切片来表示给定字符串中的一部分。
字符串切片可以通过指定起始索引和结束索引来获取字符串的一个子串。索引是从0开始计数的,起始索引是包含在结果中的,而结束索引不包含在结果中。
例如,给定字符串为"Hello, World!",我们可以使用字符串切片来表示其中的一部分。
1. 获取字符串的前三个字符:"Hel"
```python
s = "Hello, World!"
substring = s[0:3]
print(substring)
```
2. 获取字符串的第4到最后一个字符:"lo, World!"
```python
s = "Hello, World!"
substring = s[3:]
print(substring)
```
3. 获取字符串的最后五个字符:"orld!"
```python
s = "Hello, World!"
substring = s[-5:]
print(substring)
```
4. 获取字符串的某个范围内的字符:"lo, "
```python
s = "Hello, World!"
substring = s[3:8]
print(substring)
```
使用字符串切片,我们可以根据需要从给定字符串中获取所需的部分,然后进行相应的操作。在上述示例中,我们使用了不同的索引和切片操作来表示了不同的子串。
### 回答3:
一个字符串可以用Python中的字符串表示。在Python中,字符串是一系列字符的集合,可以用单引号或双引号括起来。
例如,给定字符串 "Hello, World!",可以用以下方式表示:
```python
s = "Hello, World!"
```
在上述代码中,变量 s 被赋值为字符串 "Hello, World!"。
字符串可以进行各种操作,比如连接、截取、替换等。下面是一些字符串操作的示例:
```python
s1 = "Hello"
s2 = "World"
# 字符串连接
result1 = s1 + ", " + s2 # 结果为 "Hello, World!"
# 字符串长度
length = len(s1) # 结果为 5
# 截取字符串的一部分
substring = s1[1:3] # 结果为 "el"
# 判断字符串是否包含某个子字符串
contains = "ll" in s1 # 结果为 True
# 替换字符串中的部分内容
replaced = s1.replace("l", "L") # 结果为 "HeLLo"
```
除了基本的字符串操作外,Python还提供了许多字符串处理的函数和方法。使用这些函数和方法,可以更灵活地处理字符串。
总而言之,给定一个字符串,可以使用Python的字符串表示方法来表示,并可以进行各种字符串操作和处理。
相关推荐
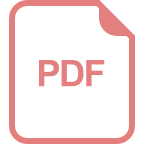
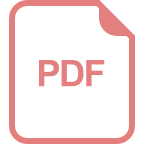
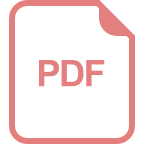












