编写函数求给定字符串中数字字符的个数在主函数中输入字符串及输出统计的个数
时间: 2024-05-08 10:22:29 浏览: 112
以下是 Python 的示例代码:
```python
def count_digits(s):
count = 0
for c in s:
if c.isdigit():
count += 1
return count
# 示例用法
s = input("请输入一个字符串:")
count = count_digits(s)
print("字符串中数字字符的个数为:", count)
```
解释一下代码:
- `count_digits` 函数接受一个字符串参数 `s`,用 `count` 变量来记录其中数字字符的数量。
- 使用 `for` 循环遍历字符串中的每个字符,对于每个字符,使用 `isdigit()` 方法来判断是否是数字字符,如果是,则将计数器 `count` 加 1。
- 循环结束后,返回数字字符的数量。
- 在主函数中,首先让用户输入一个字符串,然后调用 `count_digits` 函数来统计其中数字字符的数量,并输出结果。
注意,这里的示例代码假设输入的字符串只包含 ASCII 编码下的数字字符。如果要处理 Unicode 编码下的数字字符,需要使用 `isnumeric()` 方法来判断。
相关问题
编写函数,求给定字符串中数字字符的个数,在主函数中输入字符串及输出统计的个数。
以下是 Python 代码实现:
```python
def count_digits(s):
count = 0
for c in s:
if c.isdigit():
count += 1
return count
if __name__ == '__main__':
s = input('请输入字符串:')
print('数字字符的个数为:', count_digits(s))
```
在主函数中,我们可以通过 `input` 函数获取用户输入的字符串,然后调用 `count_digits` 函数统计数字字符的个数并输出。`count_digits` 函数中,我们遍历字符串中的每个字符,如果该字符是数字字符,就将计数器加 1,最后返回计数器的值。
c语言编写函数,求给定字符串中数字字符的个数,在主函数中输入字符串及输出统计的个数。
可以编写一个函数,接收一个字符串作为参数,然后遍历字符串,统计其中数字字符的个数,最后返回统计结果。以下是示例代码:
```c
#include <stdio.h>
int count_digits(char *str) {
int count = 0;
while (*str) {
if (*str >= '0' && *str <= '9') {
count++;
}
str++;
}
return count;
}
int main() {
char str[100];
printf("请输入字符串:");
scanf("%s", str);
int count = count_digits(str);
printf("数字字符的个数为:%d\n", count);
return 0;
}
```
首先,`count_digits` 函数接收一个指向字符串的指针作为参数。然后,通过 `while` 循环遍历字符串,每次判断当前字符是否为数字字符(即 ASCII 码值在 `'0'` 和 `'9'` 之间),如果是,则将计数器 `count` 加 1。最后,函数返回计数器的值。
在主函数中,首先定义一个字符数组 `str`,用于存储输入的字符串。然后,通过 `scanf` 函数读取用户输入的字符串。接着,调用 `count_digits` 函数统计其中数字字符的个数,并将结果存储在变量 `count` 中。最后,使用 `printf` 函数输出统计结果。
阅读全文
相关推荐
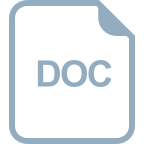
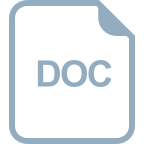
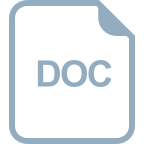












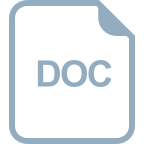
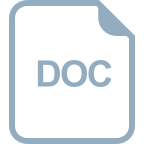