AES 加密解密算法c++实现
时间: 2023-09-19 15:09:19 浏览: 140
以下是一个简单的使用 AES 加密解密算法的 C 代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
void encrypt(unsigned char *plaintext, int plaintext_len, unsigned char *key,
unsigned char *iv, unsigned char *ciphertext)
{
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_cbc_encrypt(plaintext, ciphertext, plaintext_len, &aes_key, iv, AES_ENCRYPT);
}
void decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key,
unsigned char *iv, unsigned char *plaintext)
{
AES_KEY aes_key;
AES_set_decrypt_key(key, 128, &aes_key);
AES_cbc_encrypt(ciphertext, plaintext, ciphertext_len, &aes_key, iv, AES_DECRYPT);
}
int main()
{
unsigned char key[] = "0123456789abcdef";
unsigned char iv[] = "0123456789abcdef";
unsigned char plaintext[] = "Hello, world!";
int plaintext_len = strlen((char *)plaintext) + 1;
int ciphertext_len = ((plaintext_len - 1) / AES_BLOCK_SIZE + 1) * AES_BLOCK_SIZE;
unsigned char *ciphertext = malloc(ciphertext_len);
unsigned char *decryptedtext = malloc(ciphertext_len);
encrypt(plaintext, plaintext_len, key, iv, ciphertext);
decrypt(ciphertext, ciphertext_len, key, iv, decryptedtext);
printf("Original message: %s\n", plaintext);
printf("Encrypted message: ");
for (int i=0; i<ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
printf("Decrypted message: %s\n", decryptedtext);
free(ciphertext);
free(decryptedtext);
return 0;
}
```
在这个示例中,我们使用了 OpenSSL 库中的 AES 加密解密函数。首先,我们定义了一个加密函数和一个解密函数,分别使用 AES_set_encrypt_key 和 AES_set_decrypt_key 函数设置密钥,使用 AES_cbc_encrypt 函数加密或解密数据。然后,在 main 函数中,我们定义了一个密钥和一个初始化向量,以及要加密的明文和密文的长度。我们使用 malloc 函数动态分配了足够的空间来存储加密后的密文和解密后的明文。接下来,我们调用 encrypt 函数对明文进行加密,然后调用 decrypt 函数对密文进行解密。最后,我们输出原始消息、加密消息和解密消息,然后释放动态分配的内存。
阅读全文
相关推荐
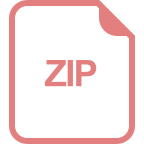
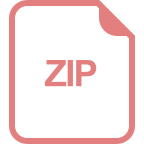
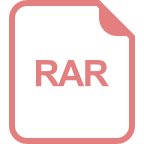
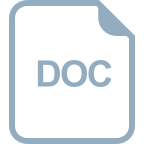
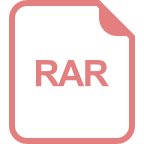
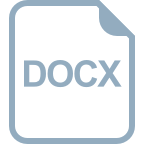
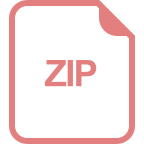
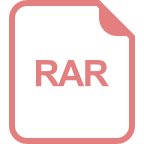
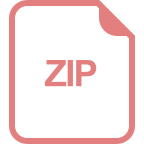
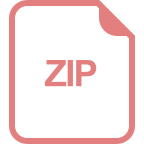
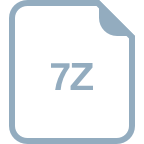
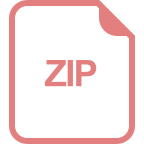
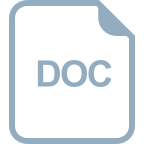
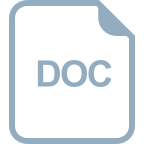
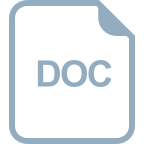

