C++ aes加密解密算法
时间: 2024-08-16 12:08:45 浏览: 33
C++ 中的 AES(Advanced Encryption Standard)加密解密通常涉及使用 OpenSSL 库提供的功能,因为 OpenSSL 提供了对 AES 的标准支持。AES是一种块密码算法,常用于数据加密,其常见的工作模式包括ECB(电子编码书),CBC( chaining block cipher mode)等。
以下是使用 OpenSSL 进行 AES 加密和解密的基本步骤:
**加密(Encrypting):**
```cpp
#include <openssl/aes.h>
// ...
std::string key = "your_secret_key";
std::string plaintext = "your_message_to_encrypt";
unsigned char iv[AES_BLOCK_SIZE] = {0}; // 初始化向量 (IV),可以随机生成并保持一致
AES_KEY aes_key;
if (!AES_set_encrypt_key(reinterpret_cast<const unsigned char*>(key.c_str()), key.length() * 8, &aes_key)) {
// 错误处理...
}
size_t ciphertext_len = AES_BLOCK_SIZE + (plaintext.size() % AES_BLOCK_SIZE);
std::vector<unsigned char> ciphertext(ciphertext_len, 0);
int result = AES_cbc_encrypt(reinterpret_cast<const unsigned char*>(plaintext.c_str()), ciphertext.data(), plaintext.size(), &aes_key, iv, AES_ENCRYPT);
if (result != AES_SUCCESS) {
// 错误处理...
}
// 将结果转换成字符串或其他形式存储
```
**解密(Decrypting):**
```cpp
std::vector<unsigned char> decrypted_data(ciphertext_len, 0);
AES_decrypt(ciphertext.data(), decrypted_data.data(), plaintext.size(), &aes_key, iv, AES_DECRYPT);
std::string decrypted_text(reinterpret_cast<char*>(decrypted_data.data()), plaintext.size());
```
相关推荐
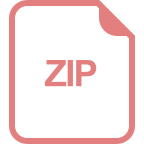
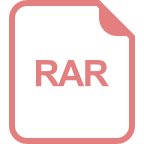
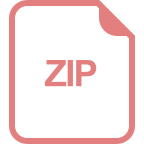














