基于tensorflow2.5,使用随机池化和L2池化替换CBAM注意力机制模块里的池化操作,并给出使用示例
时间: 2024-05-02 10:17:41 浏览: 15
首先,我们需要定义随机池化和L2池化操作。随机池化操作可以通过在输入张量上进行随机采样并对采样结果进行池化来实现。L2池化操作可以通过对输入张量中每个通道的L2范数进行池化来实现。具体实现如下:
```python
import tensorflow as tf
class RandomPooling(tf.keras.layers.Layer):
def __init__(self):
super(RandomPooling, self).__init__()
def call(self, inputs):
# 获取输入张量的形状
input_shape = tf.shape(inputs)
# 随机采样
sampled_indices = tf.random.uniform([input_shape[0], 1], maxval=input_shape[1], dtype=tf.int32)
sampled_inputs = tf.gather_nd(inputs, tf.expand_dims(sampled_indices, axis=-1), batch_dims=1)
# 池化
pooled_inputs = tf.math.reduce_max(sampled_inputs, axis=1)
return pooled_inputs
class L2Pooling(tf.keras.layers.Layer):
def __init__(self):
super(L2Pooling, self).__init__()
def call(self, inputs):
# 计算L2范数
norm = tf.norm(inputs, axis=-1, keepdims=True)
# 池化
pooled_inputs = tf.math.reduce_max(norm, axis=1)
return pooled_inputs
```
接下来,我们需要定义一个新的注意力机制模块,使用上述池化操作替换掉原先的池化操作。具体实现如下:
```python
class NewCBAM(tf.keras.layers.Layer):
def __init__(self, reduction_ratio=16):
super(NewCBAM, self).__init__()
self.reduction_ratio = reduction_ratio
# 定义全局平均池化层
self.global_avg_pooling = tf.keras.layers.GlobalAveragePooling2D()
# 定义全局最大池化层
self.global_max_pooling = tf.keras.layers.GlobalMaxPooling2D()
# 定义随机池化层
self.random_pooling = RandomPooling()
# 定义L2池化层
self.l2_pooling = L2Pooling()
# 定义两个全连接层,用于计算通道注意力和空间注意力
self.channel_dense1 = tf.keras.layers.Dense(units=self.reduction_ratio, activation='relu')
self.channel_dense2 = tf.keras.layers.Dense(units=inputs_shape[-1], activation='sigmoid')
self.spatial_dense1 = tf.keras.layers.Dense(units=self.reduction_ratio, activation='relu')
self.spatial_dense2 = tf.keras.layers.Dense(units=2, activation='sigmoid')
def call(self, inputs):
# 计算通道注意力
channel_avg_pooling = self.global_avg_pooling(inputs)
channel_max_pooling = self.global_max_pooling(inputs)
channel_features = tf.concat([channel_avg_pooling, channel_max_pooling], axis=-1)
channel_attention = self.channel_dense1(channel_features)
channel_attention = self.channel_dense2(channel_attention)
# 计算空间注意力
spatial_attention = self.l2_pooling(inputs)
spatial_attention = self.spatial_dense1(spatial_attention)
spatial_attention = self.spatial_dense2(spatial_attention)
# 将通道注意力和空间注意力相乘
attention = tf.expand_dims(channel_attention, axis=1) * tf.expand_dims(spatial_attention, axis=-1)
# 对注意力加权
weighted_inputs = inputs * attention
return weighted_inputs
```
最后,我们给出使用示例。假设我们有一个输入张量`x`,它的形状为`(batch_size, height, width, channels)`,我们可以使用新的注意力机制模块对它进行处理:
```python
# 定义一个新的CBAM模块
cbam = NewCBAM(reduction_ratio=16)
# 对输入张量进行处理
weighted_inputs = cbam(x)
```
相关推荐
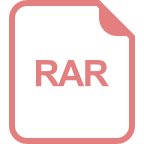














