Java将文件里json内容替换成另一个数据格式
时间: 2023-11-22 22:56:00 浏览: 42
你可以使用Java中的JSON库(例如Jackson或Gson)读取JSON文件中的内容,然后将其转换为Java对象。接下来,你可以使用Java代码来修改Java对象的属性值,然后将其转换回JSON格式并将其写回文件中。
下面是一个基本的示例代码,假设你的JSON文件名为"data.json",它包含以下内容:
```
{
"name": "John",
"age": 30,
"city": "New York"
}
```
你想要将其替换为以下内容:
```
{
"firstName": "John",
"lastName": "Doe",
"age": 35,
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": "12345"
}
}
```
你可以使用以下代码:
```java
import java.io.File;
import java.io.IOException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
public class JSONFileConverter {
public static void main(String[] args) {
try {
// 读取JSON文件
ObjectMapper objectMapper = new ObjectMapper();
ObjectNode node = (ObjectNode) objectMapper.readTree(new File("data.json"));
// 修改属性值
node.put("firstName", "John");
node.put("lastName", "Doe");
node.put("age", 35);
ObjectNode addressNode = objectMapper.createObjectNode();
addressNode.put("street", "123 Main St");
addressNode.put("city", "Anytown");
addressNode.put("state", "CA");
addressNode.put("zip", "12345");
node.set("address", addressNode);
// 将修改后的内容写回文件
objectMapper.writeValue(new File("data.json"), node);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个代码将"data.json"文件中的内容替换为上面所示的新内容。你可以根据自己的需求修改代码。
相关推荐
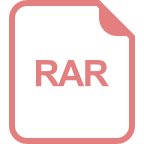
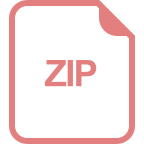
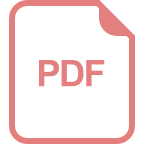
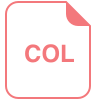










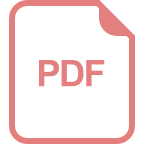
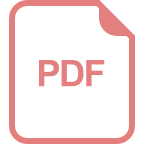
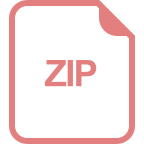
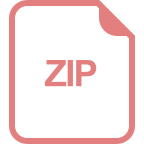