火车订票系统的C语言代码
时间: 2023-03-12 07:44:44 浏览: 108
我不知道具体的C语言代码,但是可以告诉你大致的实现步骤: 1. 定义火车订票系统的相关数据结构;2. 编写函数来实现订票系统的相关功能;3. 编写main函数来调用之前定义的函数;4. 测试程序,修正错误;5. 编译并运行程序。
相关问题
c语言实现火车票订票系统
好的,下面我会给你提供一个简单的 C 语言实现火车票订票系统的示例代码,帮助你更加深入地理解实现过程。
首先,我们需要定义几个结构体来存储用户、车次和订单信息:
```c
typedef struct {
char username[20]; // 用户名
char password[20]; // 密码
char name[20]; // 姓名
int age; // 年龄
char phone[20]; // 联系电话
} User;
typedef struct {
char train_no[20]; // 车次编号
char from[20]; // 出发地
char to[20]; // 目的地
char departure[20]; // 出发时间
int first_class; // 一等座数量
int second_class; // 二等座数量
} Train;
typedef struct {
char username[20]; // 用户名
char train_no[20]; // 车次编号
char departure[20]; // 出发时间
char seat_type[20]; // 座位类型
int seat_number; // 座位号
int price; // 订单金额
int status; // 订单状态(0:未支付,1:已支付)
} Order;
```
接下来,我们需要实现一些函数来完成用户登录/注册、车次查询、座位选择、订单生成和订单查询等功能:
```c
// 用户登录函数
int login() {
char username[20], password[20];
printf("请输入用户名:");
scanf("%s", username);
printf("请输入密码:");
scanf("%s", password);
// TODO: 从文件中读取用户信息验证登录
return 0;
}
// 用户注册函数
int register_user() {
User user;
printf("请输入用户名:");
scanf("%s", user.username);
printf("请输入密码:");
scanf("%s", user.password);
printf("请输入姓名:");
scanf("%s", user.name);
printf("请输入年龄:");
scanf("%d", &user.age);
printf("请输入联系电话:");
scanf("%s", user.phone);
// TODO: 将用户信息保存到文件中
return 0;
}
// 车次查询函数
int query_train() {
char from[20], to[20], departure[20];
printf("请输入出发地:");
scanf("%s", from);
printf("请输入目的地:");
scanf("%s", to);
printf("请输入出发时间:");
scanf("%s", departure);
// TODO: 从文件中读取符合条件的车次信息并输出
return 0;
}
// 座位选择函数
int select_seat() {
char train_no[20], departure[20], seat_type[20];
int seat_number;
printf("请输入车次编号:");
scanf("%s", train_no);
printf("请输入出发时间:");
scanf("%s", departure);
printf("请输入座位类型(1:一等座,2:二等座):");
scanf("%s", seat_type);
printf("请输入座位号:");
scanf("%d", &seat_number);
// TODO: 判断座位是否可选,如果可选则将座位状态更新到文件中
return 0;
}
// 订单生成函数
int create_order() {
char username[20], train_no[20], departure[20], seat_type[20];
int seat_number;
printf("请输入用户名:");
scanf("%s", username);
printf("请输入车次编号:");
scanf("%s", train_no);
printf("请输入出发时间:");
scanf("%s", departure);
printf("请输入座位类型(1:一等座,2:二等座):");
scanf("%s", seat_type);
printf("请输入座位号:");
scanf("%d", &seat_number);
// TODO: 生成订单并将订单信息保存到文件中
return 0;
}
// 订单查询函数
int query_order() {
char username[20];
printf("请输入用户名:");
scanf("%s", username);
// TODO: 从文件中读取符合条件的订单信息并输出
return 0;
}
```
最后,我们可以在主函数中实现一个简单的菜单来让用户选择需要执行的功能:
```c
int main() {
int choice;
do {
printf("请选择功能:\n");
printf("1. 用户登录\n");
printf("2. 用户注册\n");
printf("3. 车次查询\n");
printf("4. 座位选择\n");
printf("5. 订单生成\n");
printf("6. 订单查询\n");
printf("0. 退出\n");
scanf("%d", &choice);
switch (choice) {
case 1:
login();
break;
case 2:
register_user();
break;
case 3:
query_train();
break;
case 4:
select_seat();
break;
case 5:
create_order();
break;
case 6:
query_order();
break;
case 0:
printf("谢谢使用!\n");
break;
default:
printf("请输入正确的选项!\n");
break;
}
} while (choice != 0);
return 0;
}
```
这样,我们就完成了一个简单的 C 语言实现火车票订票系统的示例代码。当然,这只是一个简单的示例,实际开发中还需要考虑很多实际问题,比如并发访问、安全性等。但是这个示例可以帮助你了解如何使用 C 语言来开发一个火车票订票系统。
用c语言写火车订票系统项目
好的,火车订票系统是个比较复杂的项目,需要考虑到多个方面。以下是一个基本的 C 语言代码框架,可以作为你编写火车订票系统项目的起点:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_TRAINS 100
#define MAX_SEATS 100
struct train {
char name[50];
int num_seats;
int seats[MAX_SEATS];
};
struct train trains[MAX_TRAINS];
int num_trains = 0;
void add_train(char *name, int num_seats) {
struct train *t = &trains[num_trains++];
strncpy(t->name, name, sizeof(t->name));
t->num_seats = num_seats;
for (int i = 0; i < num_seats; i++) {
t->seats[i] = 0;
}
}
void list_trains() {
printf("Available trains:\n");
for (int i = 0; i < num_trains; i++) {
printf("%d. %s (%d seats)\n", i+1, trains[i].name, trains[i].num_seats);
}
}
void book_seat(int train_num, int seat_num) {
struct train *t = &trains[train_num-1];
if (seat_num < 1 || seat_num > t->num_seats) {
printf("Invalid seat number\n");
return;
}
if (t->seats[seat_num-1] != 0) {
printf("Seat already booked\n");
return;
}
t->seats[seat_num-1] = 1;
printf("Seat %d booked on train %d (%s)\n", seat_num, train_num, t->name);
}
int main() {
add_train("Train A", 5);
add_train("Train B", 10);
add_train("Train C", 20);
while (1) {
printf("\n");
list_trains();
printf("Enter train number (0 to exit): ");
int train_num;
scanf("%d", &train_num);
if (train_num == 0) {
break;
}
if (train_num < 1 || train_num > num_trains) {
printf("Invalid train number\n");
continue;
}
printf("Enter seat number: ");
int seat_num;
scanf("%d", &seat_num);
book_seat(train_num, seat_num);
}
printf("Goodbye!\n");
return 0;
}
```
这个代码框架包括了以下功能:
- 定义了一个 `struct train` 结构体来表示火车信息,包括火车名称、座位数和座位状态。
- 定义了一个 `add_train` 函数来添加新的火车信息。
- 定义了一个 `list_trains` 函数来列出所有可用的火车。
- 定义了一个 `book_seat` 函数来预定火车座位。
- 在 `main` 函数中,首先添加了一些测试用的火车信息,然后进入一个循环,让用户选择火车和座位号来预定座位。
当然,这只是一个基本的框架,你需要根据你的具体需求来进行修改和扩展。
相关推荐
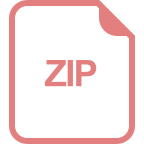
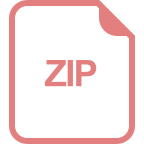










