用java写一个贪食蛇游戏
时间: 2023-12-19 16:04:52 浏览: 79
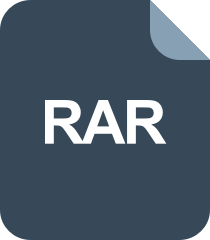
用java写的贪吃蛇游戏
以下是一个简单的贪食蛇游戏,使用Java编写。它包括一个游戏循环,可以处理用户输入,更新游戏状态并绘制游戏画面。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.*;
public class SnakeGame extends JFrame implements ActionListener {
private static final int WIDTH = 500;
private static final int HEIGHT = 500;
private static final int DOT_SIZE = 20;
private static final int ALL_DOTS = (WIDTH * HEIGHT) / (DOT_SIZE * DOT_SIZE);
private static final int RAND_POS = WIDTH / DOT_SIZE;
private static final int DELAY = 100;
private ArrayList<Point> snake;
private Point food;
private Timer timer;
private boolean inGame;
private boolean leftDirection;
private boolean rightDirection;
private boolean upDirection;
private boolean downDirection;
public SnakeGame() {
setTitle("Snake Game");
setSize(WIDTH, HEIGHT);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setResizable(false);
setVisible(true);
addKeyListener(new TAdapter());
snake = new ArrayList<Point>();
inGame = true;
leftDirection = false;
rightDirection = true;
upDirection = false;
downDirection = false;
initGame();
}
public void initGame() {
snake.clear();
snake.add(new Point(100, 100));
snake.add(new Point(80, 100));
snake.add(new Point(60, 100));
generateFood();
timer = new Timer(DELAY, this);
timer.start();
}
public void generateFood() {
int x = (int) (Math.random() * RAND_POS) * DOT_SIZE;
int y = (int) (Math.random() * RAND_POS) * DOT_SIZE;
food = new Point(x, y);
}
public void checkFood() {
if (snake.get(0).equals(food)) {
snake.add(0, new Point(food));
generateFood();
}
}
public void checkCollision() {
for (int i = 1; i < snake.size(); i++) {
if (snake.get(0).equals(snake.get(i))) {
inGame = false;
}
}
if (snake.get(0).x < 0 || snake.get(0).x >= WIDTH ||
snake.get(0).y < 0 || snake.get(0).y >= HEIGHT) {
inGame = false;
}
if (!inGame) {
timer.stop();
}
}
public void move() {
for (int i = snake.size() - 1; i > 0; i--) {
snake.set(i, new Point(snake.get(i-1)));
}
if (leftDirection) {
snake.get(0).x -= DOT_SIZE;
} else if (rightDirection) {
snake.get(0).x += DOT_SIZE;
} else if (upDirection) {
snake.get(0).y -= DOT_SIZE;
} else if (downDirection) {
snake.get(0).y += DOT_SIZE;
}
}
public void paint(Graphics g) {
super.paint(g);
if (inGame) {
g.setColor(Color.RED);
g.fillOval(food.x, food.y, DOT_SIZE, DOT_SIZE);
g.setColor(Color.BLACK);
for (Point p : snake) {
g.fillOval(p.x, p.y, DOT_SIZE, DOT_SIZE);
}
Toolkit.getDefaultToolkit().sync();
} else {
gameOver(g);
}
}
public void gameOver(Graphics g) {
String msg = "Game Over";
Font font = new Font("Arial", Font.BOLD, 16);
FontMetrics metrics = getFontMetrics(font);
g.setColor(Color.BLACK);
g.setFont(font);
g.drawString(msg, (WIDTH - metrics.stringWidth(msg)) / 2, HEIGHT / 2);
}
public void actionPerformed(ActionEvent e) {
if (inGame) {
checkFood();
checkCollision();
move();
repaint();
}
}
private class TAdapter extends KeyAdapter {
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_LEFT && !rightDirection) {
leftDirection = true;
upDirection = false;
downDirection = false;
} else if (key == KeyEvent.VK_RIGHT && !leftDirection) {
rightDirection = true;
upDirection = false;
downDirection = false;
} else if (key == KeyEvent.VK_UP && !downDirection) {
upDirection = true;
leftDirection = false;
rightDirection = false;
} else if (key == KeyEvent.VK_DOWN && !upDirection) {
downDirection = true;
leftDirection = false;
rightDirection = false;
}
}
}
public static void main(String[] args) {
new SnakeGame();
}
}
```
这个游戏使用Java Swing库来处理窗口和绘制。它使用一个ArrayList来跟踪蛇的身体,一个Point来表示食物的位置,以及一些布尔变量来跟踪蛇的方向。游戏循环在ActionListener中实现,并在每个时钟周期中更新游戏状态并重新绘制游戏画面。键盘事件处理程序允许玩家使用箭头键来控制蛇的移动。
阅读全文
相关推荐
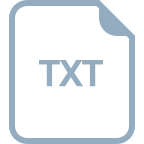
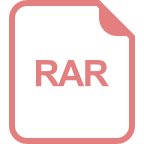
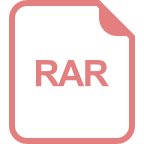
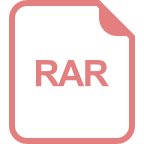
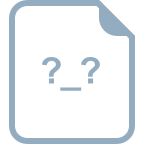
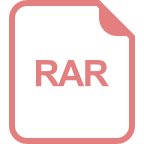
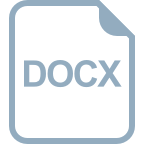
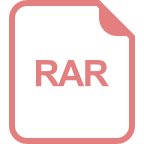
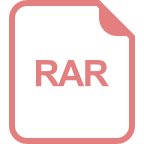
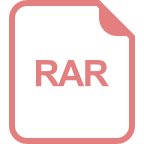
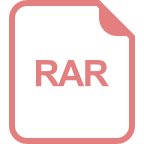
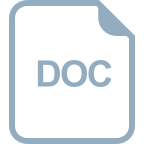
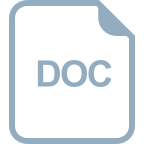

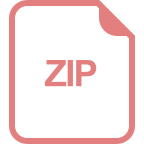