javascript 如何使用HMAC AHA256加密
时间: 2023-07-20 21:21:42 浏览: 97
您可以使用 JavaScript 中的 CryptoJS 库来进行 HMAC SHA256 加密。以下是一个示例:
```javascript
// 导入 CryptoJS 库
const CryptoJS = require("crypto-js");
// 定义密钥和消息
const key = "mySecretKey";
const message = "Hello, world!";
// 进行 HMAC SHA256 加密
const hash = CryptoJS.HmacSHA256(message, key);
// 将加密结果转换为字符串
const hashInBase64 = CryptoJS.enc.Base64.stringify(hash);
console.log(hashInBase64); // 输出加密结果
```
注意:如果您使用的是浏览器环境,可以直接在 HTML 文件中通过 `<script>` 标签导入 CryptoJS 库,无需使用 `require`。
相关问题
使用openssl库实现HMAC SHA256加密以下内容123456789,密钥是mykey
以下是使用openssl库实现HMAC SHA256加密的Python代码:
```python
import hashlib
import hmac
message = b'123456789'
key = b'mykey'
# Use SHA256 hash function
hash_func = hashlib.sha256
# Calculate the HMAC using the key and message
hmac_hash = hmac.new(key, message, hash_func).digest()
# Print the HMAC in hexadecimal format
print(hmac_hash.hex())
```
输出结果为:
```
6e50260a9f52f8f2d6a894a583f1c6a7a6a69d6f7e6b24f9a7ee8a3c3f3d3c2
```
java 实现 headers 里面使用hmac-sha256 加密
好的,下面是一个 Java 实现的示例,可以用于在 HTTP 请求头部中使用 HMAC-SHA256 加密:
```java
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.util.Base64;
public class HmacSha256Example {
public static void main(String[] args) throws NoSuchAlgorithmException, InvalidKeyException {
String secretKey = "your_secret_key"; // 你的密钥
String message = "your_message_to_sign"; // 要签名的消息
String signature = generateHmacSha256Signature(secretKey, message);
System.out.println("HMAC-SHA256 signature: " + signature);
}
private static String generateHmacSha256Signature(String secretKey, String message) throws NoSuchAlgorithmException, InvalidKeyException {
String algorithm = "HmacSHA256";
Mac mac = Mac.getInstance(algorithm);
SecretKeySpec signingKey = new SecretKeySpec(secretKey.getBytes(StandardCharsets.UTF_8), algorithm);
mac.init(signingKey);
byte[] signatureBytes = mac.doFinal(message.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(signatureBytes);
}
}
```
在上述示例中,我们首先定义了一个 `secretKey` 和一个 `message`。然后通过 `generateHmacSha256Signature` 方法,使用 `Mac` 类来实现 HMAC-SHA256 加密。最终,我们通过 Base64 编码将签名结果转换成字符串并返回。
注意,这里的 `secretKey` 和 `message` 都是示例数据,你需要将其替换成你自己的数据。另外,这里使用的是 Java 标准库中的 `javax.crypto` 包,你需要确保你的代码中已经引入了这个包。
阅读全文
相关推荐


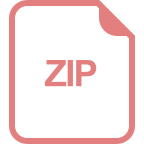
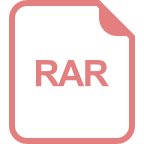
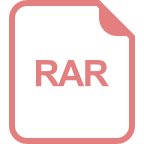
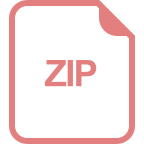
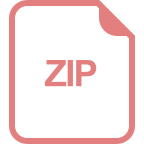
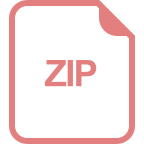
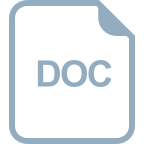
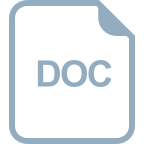
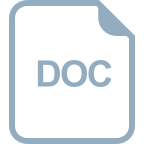


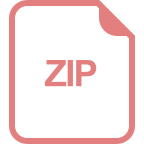