字节流和字符流的区别是什么?如何实现字节流与字符流的转换? 简述对象序列化和反序列化及其实现方法,并编写程序实现简单的序列化与反序列化。
时间: 2023-06-27 22:06:46 浏览: 89
字节流和字符流的区别在于,字节流以字节为单位进行读写操作,而字符流以字符为单位进行读写操作。字节流能够处理所有类型的数据,包括文本、图像、音频等,但是对于文本处理来说,字节流需要考虑编码问题。而字符流则专门用于处理文本数据,可以自动处理编码相关的问题。
实现字节流与字符流的转换可以使用InputStreamReader和OutputStreamWriter类,将字节流转换为字符流,或者将字符流转换为字节流。具体代码如下:
```java
// 将字节流转换为字符流
InputStream inputStream = new FileInputStream("input.txt");
Reader reader = new InputStreamReader(inputStream, "UTF-8");
// 将字符流转换为字节流
OutputStream outputStream = new FileOutputStream("output.txt");
Writer writer = new OutputStreamWriter(outputStream, "UTF-8");
```
对象序列化是指将对象转换为字节序列的过程,反序列化则是将字节序列转换为对象的过程。Java提供了ObjectOutputStream和ObjectInputStream类来实现对象的序列化和反序列化。具体代码如下:
```java
import java.io.*;
public class SerializationDemo {
public static void main(String[] args) {
// 序列化对象
try {
FileOutputStream fileOut = new FileOutputStream("employee.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
Employee e = new Employee("Tom", "Developer", 10000);
out.writeObject(e);
out.close();
fileOut.close();
System.out.println("Serialized data is saved in employee.ser");
} catch (IOException i) {
i.printStackTrace();
}
// 反序列化对象
try {
FileInputStream fileIn = new FileInputStream("employee.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Employee e = (Employee) in.readObject();
in.close();
fileIn.close();
System.out.println("Deserialized data: " + e.toString());
} catch (IOException i) {
i.printStackTrace();
} catch (ClassNotFoundException c) {
System.out.println("Employee class not found");
c.printStackTrace();
}
}
}
class Employee implements Serializable {
private String name;
private String job;
private double salary;
public Employee(String name, String job, double salary) {
this.name = name;
this.job = job;
this.salary = salary;
}
public String getName() {
return name;
}
public String getJob() {
return job;
}
public double getSalary() {
return salary;
}
public String toString() {
return "Name: " + name + ", Job: " + job + ", Salary: " + salary;
}
}
```
以上代码中,Employee类实现了Serializable接口,使得其可以被序列化。在序列化过程中,使用ObjectOutputStream将Employee对象写入文件中;在反序列化过程中,使用ObjectInputStream将文件中的字节流读取出来,并将其转换为Employee对象。
相关推荐
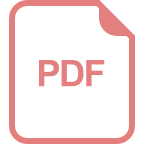
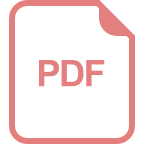
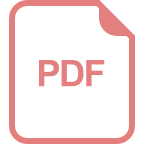














