假设线性表( a1 ,a2 ,…, an )的数据元素存储在一维数组 A[n]中,则从数组的第一个分量起,依次生成结点,并逐个插入到一个初始为“空”的链表中。请用C语言编写代码
时间: 2024-10-08 12:24:40 浏览: 54
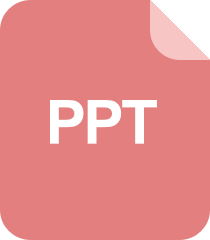
数据结构:第5章 数组和稀疏矩阵.ppt

这是一个常见的将一维数组转换为单链表的问题,我们可以使用结构体表示节点,然后遍历数组并创建新节点插入到链表中。以下是C语言的一个简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建新节点并插入链表
Node* insertInList(int value, Node* head) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 动态分配内存
if (!newNode) { // 如果内存分配失败
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = head; // 新节点的next指向当前链表头部
if (head != NULL) { // 如果链表非空,更新头节点的next
head->next = newNode;
} else { // 链表为空,直接设置头节点
head = newNode;
}
return head;
}
int main() {
const int n = 5; // 数组长度
int arr[] = {1, 2, 3, 4, 5}; // 示例数组
Node* listHead = NULL; // 初始化链表头指针
for (int i = 0; i < n; i++) {
listHead = insertInList(arr[i], listHead); // 插入每个数组元素
}
// 打印链表
Node* temp = listHead;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
return 0;
}
```
在这个例子中,`insertInList`函数负责创建新节点并将它们添加到链表中。`main`函数则用于初始化链表和数组,以及最后打印链表内容。
阅读全文
相关推荐
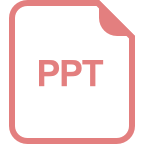
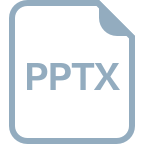










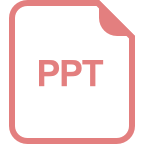
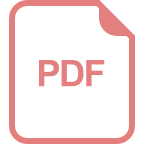
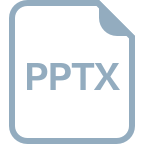
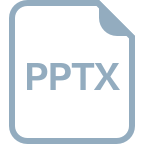
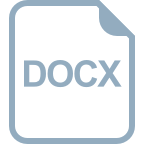
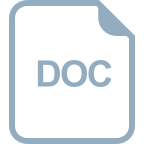