编写java代码 从阿里云OSS下载指定目录下的所有文件
时间: 2023-05-16 07:07:36 浏览: 129
您好,以下是Java代码示例,可以从阿里云OSS下载指定目录下的所有文件:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import com.aliyun.oss.ClientException;
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import com.aliyun.oss.OSSException;
import com.aliyun.oss.model.ListObjectsRequest;
import com.aliyun.oss.model.ListObjectsResult;
import com.aliyun.oss.model.OSSObject;
import com.aliyun.oss.model.ObjectListing;
public class DownloadAllFilesFromOSS {
public static void main(String[] args) {
String endpoint = "yourEndpoint";
String accessKeyId = "yourAccessKeyId";
String accessKeySecret = "yourAccessKeySecret";
String bucketName = "yourBucketName";
String prefix = "yourPrefix"; // 指定目录
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
List<String> objectKeys = new ArrayList<String>();
ObjectListing objectListing = null;
do {
ListObjectsRequest listObjectsRequest = new ListObjectsRequest(bucketName).withPrefix(prefix);
if (objectListing != null) {
listObjectsRequest.withMarker(objectListing.getNextMarker());
}
objectListing = ossClient.listObjects(listObjectsRequest);
for (OSSObject ossObject : objectListing.getObjectSummaries()) {
objectKeys.add(ossObject.getKey());
}
} while (objectListing.isTruncated());
for (String objectKey : objectKeys) {
try {
OSSObject ossObject = ossClient.getObject(bucketName, objectKey);
byte[] buffer = new byte[1024];
int len;
File file = new File(objectKey);
File parentFile = file.getParentFile();
if (!parentFile.exists()) {
parentFile.mkdirs();
}
FileOutputStream fos = new FileOutputStream(file);
while ((len = ossObject.getObjectContent().read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fos.close();
ossObject.getObjectContent().close();
} catch (OSSException oe) {
oe.printStackTrace();
} catch (ClientException ce) {
ce.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
ossClient.shutdown();
}
}
```
请将代码中的 `yourEndpoint`、`yourAccessKeyId`、`yourAccessKeySecret`、`yourBucketName`、`yourPrefix` 替换为您自己的阿里云OSS信息。
相关推荐
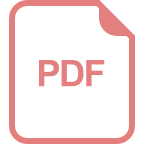
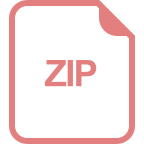














