使用XML和注解的方式分别实现SpringAOP的前置增强、后置增强、环绕增强、抛出异常增强、最终增强(编程题)60’
时间: 2023-07-10 16:05:05 浏览: 55
首先,需要在Spring配置文件中开启AOP的支持:
```xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<!-- AOP 配置 -->
<aop:aspectj-autoproxy />
<!-- 其他 Bean 的配置 -->
<!-- ... -->
</beans>
```
然后,分别使用XML和注解的方式实现各种增强:
## 前置增强
使用XML配置:
```xml
<aop:config>
<aop:aspect id="myAspect" ref="myAspectBean">
<aop:before pointcut="execution(* com.example.service.*.*(..))" method="beforeAdvice" />
</aop:aspect>
</aop:config>
```
使用注解:
```java
@Aspect
@Component
public class MyAspect {
@Before("execution(* com.example.service.*.*(..))")
public void beforeAdvice() {
// 前置增强的逻辑
}
}
```
## 后置增强
使用XML配置:
```xml
<aop:config>
<aop:aspect id="myAspect" ref="myAspectBean">
<aop:after-returning pointcut="execution(* com.example.service.*.*(..))" method="afterAdvice" />
</aop:aspect>
</aop:config>
```
使用注解:
```java
@Aspect
@Component
public class MyAspect {
@AfterReturning("execution(* com.example.service.*.*(..))")
public void afterAdvice() {
// 后置增强的逻辑
}
}
```
## 环绕增强
使用XML配置:
```xml
<aop:config>
<aop:aspect id="myAspect" ref="myAspectBean">
<aop:around pointcut="execution(* com.example.service.*.*(..))" method="aroundAdvice" />
</aop:aspect>
</aop:config>
```
使用注解:
```java
@Aspect
@Component
public class MyAspect {
@Around("execution(* com.example.service.*.*(..))")
public Object aroundAdvice(ProceedingJoinPoint joinPoint) throws Throwable {
// 环绕增强的前置逻辑
Object result = joinPoint.proceed();
// 环绕增强的后置逻辑
return result;
}
}
```
## 抛出异常增强
使用XML配置:
```xml
<aop:config>
<aop:aspect id="myAspect" ref="myAspectBean">
<aop:after-throwing pointcut="execution(* com.example.service.*.*(..))" method="afterThrowingAdvice" />
</aop:aspect>
</aop:config>
```
使用注解:
```java
@Aspect
@Component
public class MyAspect {
@AfterThrowing("execution(* com.example.service.*.*(..))")
public void afterThrowingAdvice() {
// 抛出异常增强的逻辑
}
}
```
## 最终增强
使用XML配置:
```xml
<aop:config>
<aop:aspect id="myAspect" ref="myAspectBean">
<aop:after pointcut="execution(* com.example.service.*.*(..))" method="finallyAdvice" />
</aop:aspect>
</aop:config>
```
使用注解:
```java
@Aspect
@Component
public class MyAspect {
@After("execution(* com.example.service.*.*(..))")
public void finallyAdvice() {
// 最终增强的逻辑
}
}
```
相关推荐
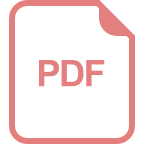














