三个大整数相加c++
时间: 2024-08-24 11:00:48 浏览: 71
在C++中,三个大整数相加的实现很简单。由于C++的基本数据类型(如int, long)都有固定的大小限制,对于超出这些限制的大整数,我们可以使用string或者vector等容器来处理,把大整数当作字符串来处理每一位。下面是一个简单的方法来实现三个大整数相加:
1. 将大整数以字符串形式输入。
2. 从字符串的最低位(即字符串的末尾)开始逐位相加,将结果存储在另一个字符串中。
3. 如果存在进位,则需要将进位加到下一位的计算中。
4. 最后,处理可能出现的最高位进位。
下面是实现这个逻辑的示例代码:
```cpp
#include <iostream>
#include <string>
#include <algorithm>
std::string addBigNumbers(const std::string& num1, const std::string& num2, const std::string& num3) {
std::string result;
int len1 = num1.size(), len2 = num2.size(), len3 = num3.size();
int maxLen = std::max({len1, len2, len3});
int carry = 0;
// Pad the shorter numbers with zeros on the left
std::string paddedNum1 = std::string(maxLen - len1, '0') + num1;
std::string paddedNum2 = std::string(maxLen - len2, '0') + num2;
std::string paddedNum3 = std::string(maxLen - len3, '0') + num3;
// Perform addition
for (int i = 0; i < maxLen; ++i) {
int sum = (paddedNum1[i] - '0') + (paddedNum2[i] - '0') + (paddedNum3[i] - '0') + carry;
carry = sum / 10;
result.push_back(sum % 10 + '0');
}
// If there is still a carry left
if (carry > 0) {
result.push_back(carry + '0');
}
// Reverse the result as we built it backwards
std::reverse(result.begin(), result.end());
return result;
}
int main() {
std::string num1, num2, num3;
std::cout << "Enter the first big number: ";
std::cin >> num1;
std::cout << "Enter the second big number: ";
std::cin >> num2;
std::cout << "Enter the third big number: ";
std::cin >> num3;
std::string sum = addBigNumbers(num1, num2, num3);
std::cout << "The sum of the three numbers is: " << sum << std::endl;
return 0;
}
```
在上述代码中,我们首先确保所有数字都被转换为同一长度(通过在较短的数字前面填充零),然后逐位进行加法运算,并处理进位。最后,如果最高位有进位,则将其添加到结果字符串的开头。
阅读全文
相关推荐
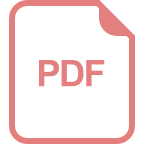
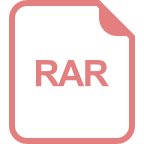
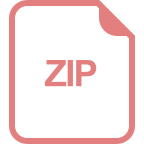











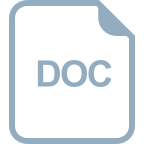



