用C语言实现:【问题描述】 回文判断:假设称正读和反读都相同的字符序列为“回文”,例如‘abba’和‘abcba’是回文,‘abcde’和‘ababab’则不是。试写一个算法判别读入的一个以‘@’结束符的字符序列是否是“回文”。 提示:利用栈和队列知识。 Copying judgment: Assuming that the character sequence known as the same reading and anti-reading is "replying", such as 'abba' and 'abcba' are repayment, 'Abcde' and 'Ababab' are not. Try to write a character sequence of an algorithm to read in the '@' ending character. Tip: Use stacks and queue knowledge. 【输入形式】 以@结尾的字符串 【输出形式】 判断结果 【样例输入】 abcdcba@ 【样例输出】 此字符串为回文
时间: 2024-01-08 20:04:53 浏览: 137
以下是C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct {
char data[MAX_SIZE];
int top;
} Stack;
typedef struct {
char data[MAX_SIZE];
int front;
int rear;
} Queue;
void InitStack(Stack *s) {
s->top = -1;
}
void Push(Stack *s, char ch) {
if (s->top == MAX_SIZE - 1) {
printf("Stack overflow!");
exit(1);
}
s->data[++s->top] = ch;
}
char Pop(Stack *s) {
if (s->top == -1) {
printf("Stack underflow!");
exit(1);
}
return s->data[s->top--];
}
void InitQueue(Queue *q) {
q->front = q->rear = 0;
}
void EnQueue(Queue *q, char ch) {
if (q->rear == MAX_SIZE - 1) {
printf("Queue overflow!");
exit(1);
}
q->data[q->rear++] = ch;
}
char DeQueue(Queue *q) {
if (q->front == q->rear) {
printf("Queue underflow!");
exit(1);
}
return q->data[q->front++];
}
int main() {
char str[MAX_SIZE];
Stack s;
Queue q;
int i, len, is_palindrome = 1;
printf("Please input a string with @ as the ending character:\n");
scanf("%s", str);
len = strlen(str);
InitStack(&s);
InitQueue(&q);
for (i = 0; i < len - 1; i++) {
Push(&s, str[i]);
EnQueue(&q, str[i]);
}
while (s.top != -1 && q.front != q.rear) {
if (Pop(&s) != DeQueue(&q)) {
is_palindrome = 0;
break;
}
}
if (is_palindrome) {
printf("This string is a palindrome.\n");
} else {
printf("This string is not a palindrome.\n");
}
return 0;
}
```
该算法使用栈和队列来实现,先将输入字符串中除了结尾的'@'之外的字符依次入栈和入队,然后依次弹出栈顶和队首的字符进行比较,如果出现不相等的情况,则说明该字符串不是回文。
阅读全文
相关推荐
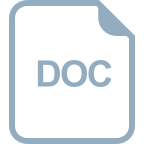
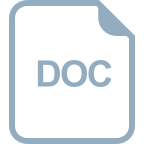
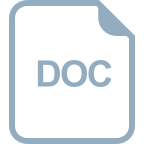














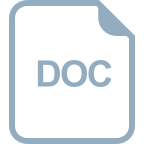
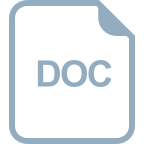