C语言数据结构和ACM2023-第四章-循环队列判断回文- LJD 【问题描述】 回文判断:假设称正读和反读都相同的字符序列为“回文”,例如‘abba’和‘abcba’是回文,‘abcde’和‘ababab’则不是。试写一个算法判别读入的一个以‘@’结束符的字符序列是否是“回文”。 提示:利用栈和队列知识。 Copying judgment: Assuming that the character sequence known as the same reading and anti-reading is "replying", such as 'abba' and 'abcba' are repayment, 'Abcde' and 'Ababab' are not. Try to write a character sequence of an algorithm to read in the '@' ending character. Tip: Use stacks and queue knowledge. 【输入形式】 以@结尾的字符串 【输出形式】 判断结果 【样例输入】 abcdcba@ 【样例输出】 此字符串为回文
时间: 2023-06-27 09:03:08 浏览: 80
以下是C语言的实现,使用循环队列和栈结构判断回文:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct {
char data[MAX_SIZE];
int front, rear;
} Queue;
typedef struct {
char data[MAX_SIZE];
int top;
} Stack;
void initQueue(Queue *q) {
q->front = q->rear = 0;
}
void initStack(Stack *s) {
s->top = -1;
}
int isEmptyQueue(Queue *q) {
return q->front == q->rear;
}
int isFullQueue(Queue *q) {
return q->front == (q->rear + 1) % MAX_SIZE;
}
int isEmptyStack(Stack *s) {
return s->top == -1;
}
int isFullStack(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void enqueue(Queue *q, char c) {
if (isFullQueue(q)) {
printf("Queue is full\n");
exit(1);
}
q->data[q->rear] = c;
q->rear = (q->rear + 1) % MAX_SIZE;
}
char dequeue(Queue *q) {
if (isEmptyQueue(q)) {
printf("Queue is empty\n");
exit(1);
}
char c = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE;
return c;
}
void push(Stack *s, char c) {
if (isFullStack(s)) {
printf("Stack is full\n");
exit(1);
}
s->data[++s->top] = c;
}
char pop(Stack *s) {
if (isEmptyStack(s)) {
printf("Stack is empty\n");
exit(1);
}
return s->data[s->top--];
}
int main() {
char str[MAX_SIZE];
printf("Input a string ending with '@': ");
scanf("%s", str);
int length = strlen(str);
if (str[length - 1] != '@') {
printf("Invalid input\n");
return 0;
}
Queue q;
Stack s;
initQueue(&q);
initStack(&s);
int i;
for (i = 0; i < length - 1; i++) {
enqueue(&q, str[i]);
push(&s, str[i]);
}
while (!isEmptyQueue(&q) && !isEmptyStack(&s)) {
if (dequeue(&q) != pop(&s)) {
printf("This string is not a palindrome\n");
return 0;
}
}
printf("This string is a palindrome\n");
return 0;
}
```
以上程序可以根据输入的字符串进行回文判断,如果是回文则输出"This string is a palindrome",否则输出"This string is not a palindrome"。
相关推荐
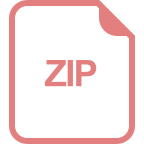














