生成一个动作类的小游戏的python代码
时间: 2024-02-23 19:03:11 浏览: 145
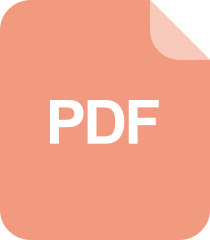
使用Python写一个小游戏
好的,以下是一个类似于“跳跳乐”的小游戏的Python代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 游戏窗口大小
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 800
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("跳跳乐")
# 加载图片
player_image = pygame.image.load("player.png").convert_alpha()
platform_image = pygame.image.load("platform.png").convert_alpha()
# 设置字体
font = pygame.font.SysFont(None, 48)
# 定义玩家类
class Player(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = player_image
self.rect = self.image.get_rect()
self.rect.centerx = SCREEN_WIDTH // 2
self.rect.bottom = SCREEN_HEIGHT - 50
self.speed = 0
def update(self):
# 根据速度更新玩家位置
self.rect.y -= self.speed
self.speed -= 1
# 玩家掉出屏幕,游戏结束
if self.rect.bottom > SCREEN_HEIGHT:
game_over()
def jump(self):
# 玩家跳跃
self.speed = 20
# 定义平台类
class Platform(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.image = platform_image
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
def update(self):
# 平台向下移动
self.rect.y -= 5
# 平台移出屏幕,重新生成平台
if self.rect.top < 0:
self.rect.bottom = SCREEN_HEIGHT
self.rect.x = random.randint(0, SCREEN_WIDTH - self.rect.width)
# 创建玩家和平台组
all_sprites = pygame.sprite.Group()
platforms = pygame.sprite.Group()
# 创建玩家
player = Player()
all_sprites.add(player)
# 创建初始平台
platform = Platform(0, SCREEN_HEIGHT - 40)
all_sprites.add(platform)
platforms.add(platform)
# 主循环
def main():
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
player.jump()
# 更新玩家和平台
all_sprites.update()
platforms.update()
# 玩家与平台碰撞检测
if player.speed < 0:
hits = pygame.sprite.spritecollide(player, platforms, False)
if hits:
player.rect.bottom = hits[0].rect.top
player.speed = 0
# 生成新平台
if len(platforms) < 5:
x = random.randint(0, SCREEN_WIDTH - platform_image.get_width())
y = random.randint(-100, -50)
platform = Platform(x, y)
all_sprites.add(platform)
platforms.add(platform)
# 绘制游戏场景
screen.fill(WHITE)
all_sprites.draw(screen)
# 显示得分
score = len(platforms) - 1
score_text = font.render("得分:%d" % score, True, RED)
screen.blit(score_text, (10, 10))
# 更新屏幕
pygame.display.update()
# 游戏结束
def game_over():
pygame.quit()
sys.exit()
# 启动游戏
main()
```
玩家需要控制角色跳跃,不断在移动的平台上跳跃,尽可能获得更高的分数。如果角色掉出屏幕,游戏结束。游戏会不断生成新的平台,玩家需要注意跳跃的时机和平台的位置。玩家得分由跳跃的平台数量决定。
阅读全文
相关推荐
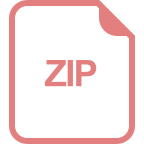
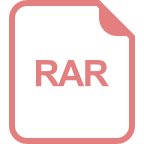
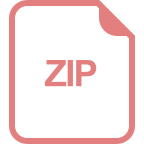
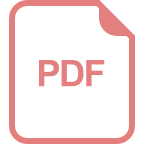
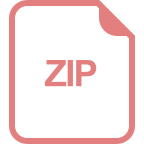
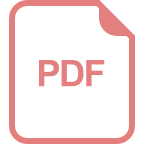
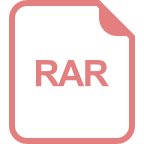
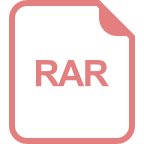
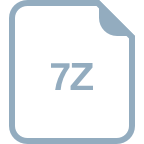
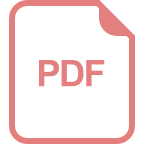
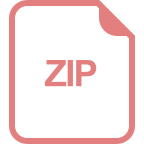
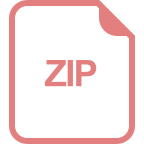
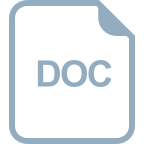
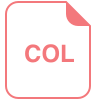
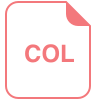
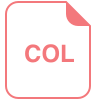